Want to build a powerful Live search box using PHP, MySQL, and Ajax?
If yes! Then this guide will help you explore how to create a powerful live search.
Our goal is to provide you with an itemized step-by-step guide so that you can create a Live search box for yourself, understand the technical aspects of building a live search feature, and also outrank other websites.
The live search feature, or AJAX search, refers to the search engine beginning to display results as you type text into the input field. You can view search results without bringing up a display page, thanks to this feature . As a result, people may locate what they’re seeking more quickly.. The development of AJAX search uses JavaScript (jQuery), MySQL, PHP, and AJAX.
Even Google, the most popular search implements the idea of Live search on its page:
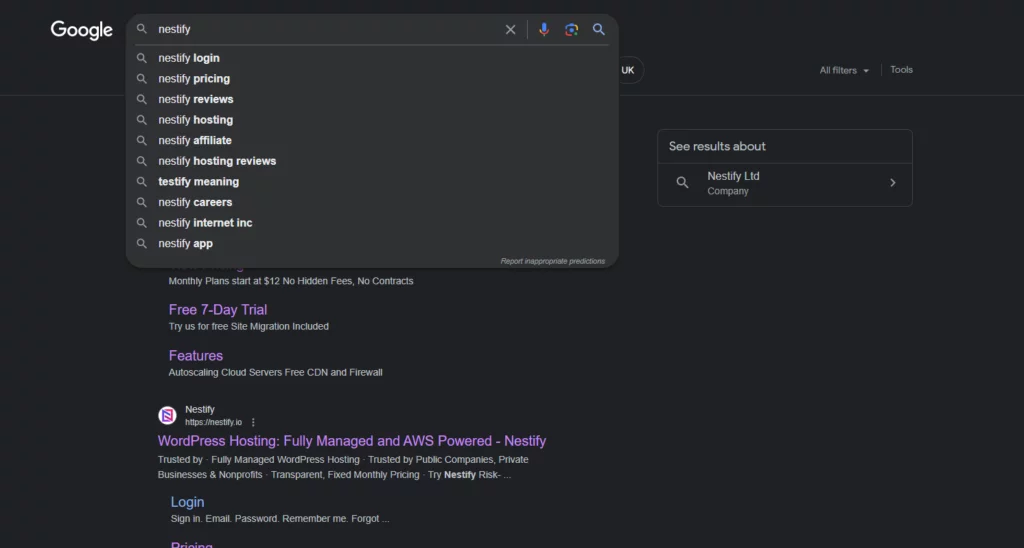
Requirement before start
- A basic understanding of HTML, CSS, PHP, MySQL, and Javascript
- A local or remote server with Apache and MySQL (either XAMPP or WAMP)
- Code editor – Downloads | Notepad++
For this article, I’m using XAMPP. On my local PC, I will first compile the script. To do this, I’ll need to link the AJAX form to a MySQL database that contains some test data.
Database Setup
After installing the XAMPP Control Panel, start Apache and MySQL.
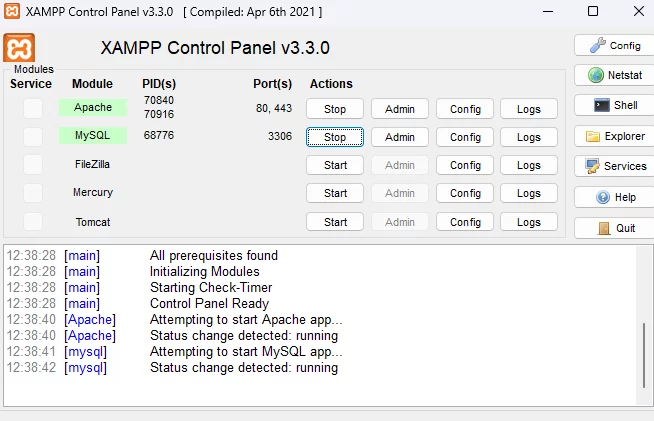
Now open the URL: https://localhost/phpmyadmin/ – Yours may be different.
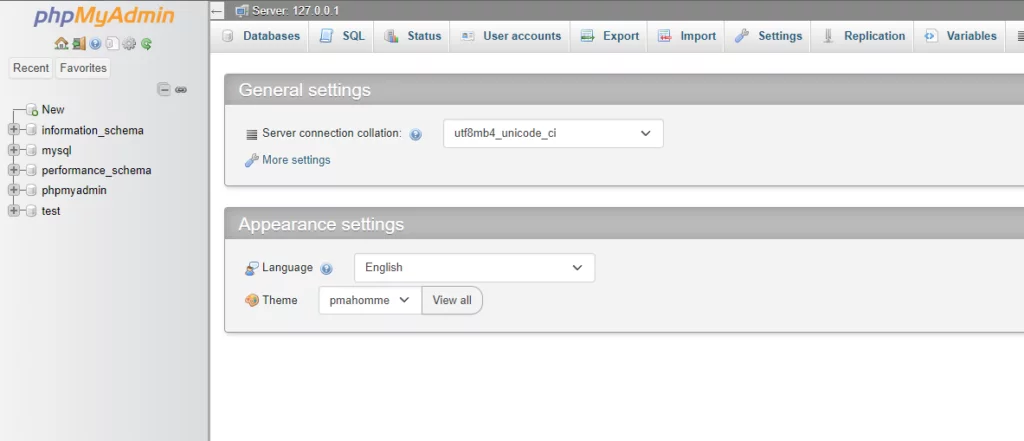
Now Click on “New”
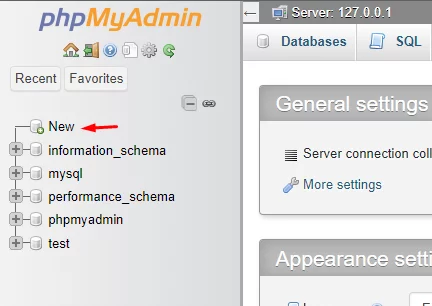
Create a database name ”Classmate” (or whatever you like to call it)
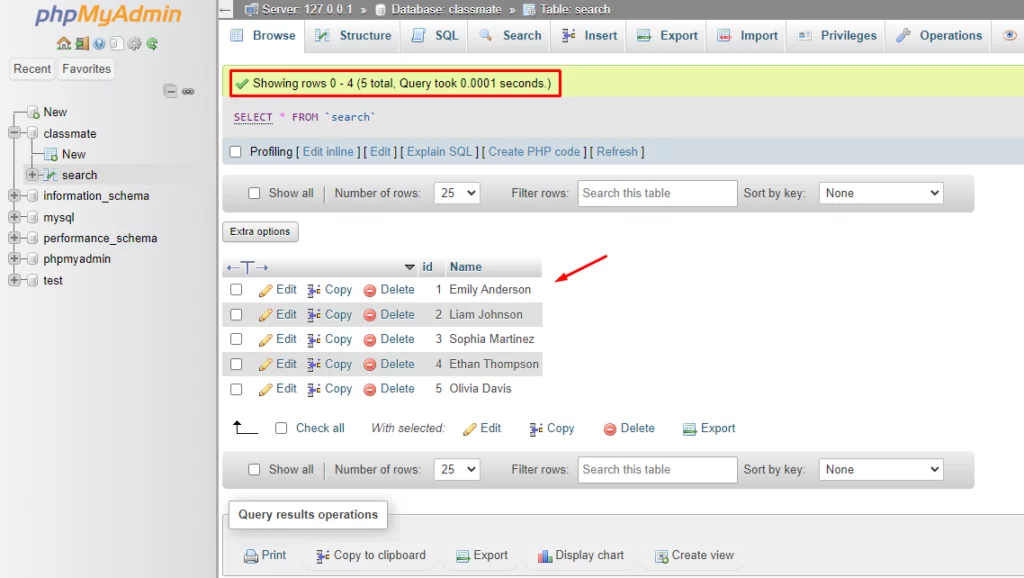
The following query should be copied and pasted to construct the table (search), column names (Id, Name), and then enter fictitious data.
CREATE TABLE search (
id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY,
Name VARCHAR(30) NOT NULL
);
INSERT INTO `search` VALUES
(1, 'Emily Anderson'),
(2, 'Liam Johnson'),
(3, 'Sophia Martinez'),
(4, 'Ethan Thompson'),
(5, 'Olivia Davis');
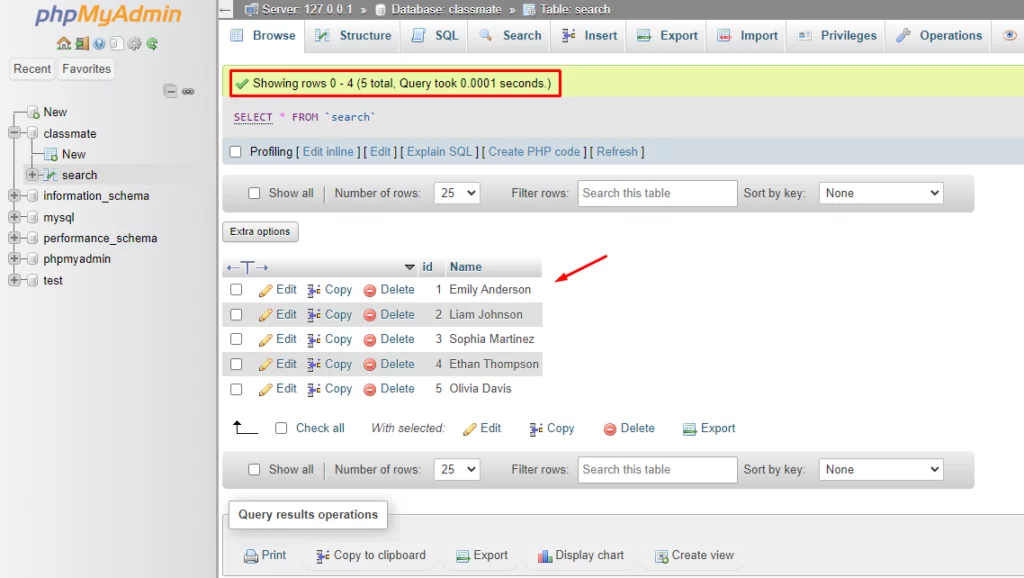
Setup Files
The following files need to be created in the htdocs folder, which are categorically located at C:/xampp/htdocs:
- search.php: This serves as the main file for the search engine, enabling user input and result display.
- db.php: This file contains the database connection details.
- ajax.php: This is the primary file responsible for generating AJAX requests to the server and retrieving results.
- script.js: Contains JavaScript functions for executing AJAX requests.
- style.css: Provides styling for the search engine.
Creating Search Form and Related Files In this step,
We will create the following files:
Search.php
Name the file search.php while creating. This is a main file of a search engine, where the user can view the result and input data. Put the code below into it by simply pasting it.
<!DOCTYPE html>
<html>
<head>
<title>Live Search using AJAX</title>
<!-- jQuery library is required. -->
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.2/jquery.min.js"></script>
<!-- Include our script file. -->
<script type="text/javascript" src="script.js"></script>
<!-- Include the CSS file. -->
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<!-- Search box. -->
<input type="text" id="search" placeholder="Search" />
<br>
<b>Example: </b><i>Emily Anderson, Liam Johnson, Sophia Martinez, Ethan Thompson, Olivia Davis</i>
<br />
<!-- Suggestions will be displayed in the following div. -->
<div id="display"></div>
</body>
</html>
db.php
This file contains the necessary details for establishing a database connection. Create a file named “db.php” and insert the following code:
<?php
// Establish the database connection.
$con = MySQLi_connect(
"localhost", // Server host name.
"root", // Database username.
"", // Database password.
"autocomplete" // Database name or any desired name.
);
// Check connection.
if (MySQLi_connect_errno()) {
echo "Failed to connect to MySQL: " . MySQLi_connect_error();
}
?>
ajax.php
This file acts as the main source for generating AJAX requests to the server and retrieving the results. Make a file called “ajax.php” and put the following code in it:
<?php
// Include the database configuration file.
include "db.php";
// Retrieve the value of the "search" variable from "script.js".
if (isset($_POST['search'])) {
// Assign the search box value to the $Name variable.
$Name = $_POST['search'];
// Define the search query.
$Query = "SELECT Name FROM search WHERE Name LIKE '%$Name%' LIMIT 5";
// Execute the query.
$ExecQuery = MySQLi_query($con, $Query);
// Create an unordered list to display the results.
echo '
<ul>
';
// Fetch the results from the database.
while ($Result = MySQLi_fetch_array($ExecQuery)) {
?>
<!-- Create list items.
Call the JavaScript function named "fill" found in "script.js".
Pass the fetched result as a parameter. -->
<li onclick='fill("<?php echo $Result['Name']; ?>")'>
<a>
<!-- Display the searched result in the search box of "search.php". -->
<?php echo $Result['Name']; ?>
</li></a>
<!-- The following PHP code is only for closing parentheses. Do not be confused. -->
<?php
}}
?>
</ul>
script.js
This file contains JavaScript functions that perform AJAX requests. Add the following code to the “script.js” file you just created:
// Retrieve the value from "ajax.php".
function fill(Value) {
// Assign the value to the "search" div in "search.php".
$('#search').val(Value);
// Hide the "display" div in "search.php".
$('#display').hide();
}
$(document).ready(function() {
// When a key is pressed in the "Search box" of "search.php", this function will be called.
$("#search").keyup(function() {
// Assign the search box value to a JavaScript variable named "name".
var name = $('#search').val();
// Validate if "name" is empty.
if (name == "") {
// Assign an empty value to the "display" div in "search.php".
$("#display").html("");
}
// If the name is not empty.
else {
// Initiate an AJAX request.
$.ajax({
// Set the AJAX type as "POST".
type: "POST",
// Send data to "ajax.php".
url: "ajax.php",
// Pass the value of "name" into the "search" variable.
data: {
search: name
},
// If a result is found, this function will be called.
success: function(html) {
// Assign the result to the "display" div in "search.php".
$("#display").html(html).show();
}
});
}
});
});
style.css
This file contains styling elements for the search engine. Add the following code to a file called “style.css“:
a:hover {
cursor: pointer;
background-color: yellow;
}
- The search engine is now ready for testing. To proceed, follow these steps:
localhost:8080/search.php
- Enter some text in the search field to perform a search in the database.
Note that as you type, the text will be sent to the script.js file, which will then forward the request to ajax.php. In ajax.php, a JavaScript function named “fill()” will process the fetched results and display them in the “display” div of the search.php file.
Conclusion
Congratulations! You have been successful in using PHP, MySQL, and AJAX to create a dynamic live search box. You now own a strong tool that can offer a flawless search experience to visitors to your website after completing this thorough guide. To make sure your website appears high on search engine result pages, keep in mind to regularly improve your content and track its performance.
FAQs on Live Search Box
Can I implement a live search box without using AJAX?
While it’s possible to create a live search box using other technologies, AJAX is the recommended approach for its seamless and real-time data fetching capabilities.
Do I need advanced programming skills to build a live search box?
Basic knowledge of PHP, MySQL, HTML, CSS, and JavaScript is required. However, following the steps provided in this article will guide you through the process.
Can I customize the appearance of the live search box to match my website’s design?
Yes, you can customize the HTML and CSS of the live search box to ensure it aligns with your website’s branding and design.
Is it possible to include additional search features, such as filters or sorting options?
Absolutely! Once you have the core live search functionality implemented, you can expand upon it and add more advanced search features based on your specific requirements.
Will the live search box work on mobile devices?
Yes, by ensuring mobile responsiveness in your design and development process, the live search box will function seamlessly on all devices, including mobile phones and tablets.