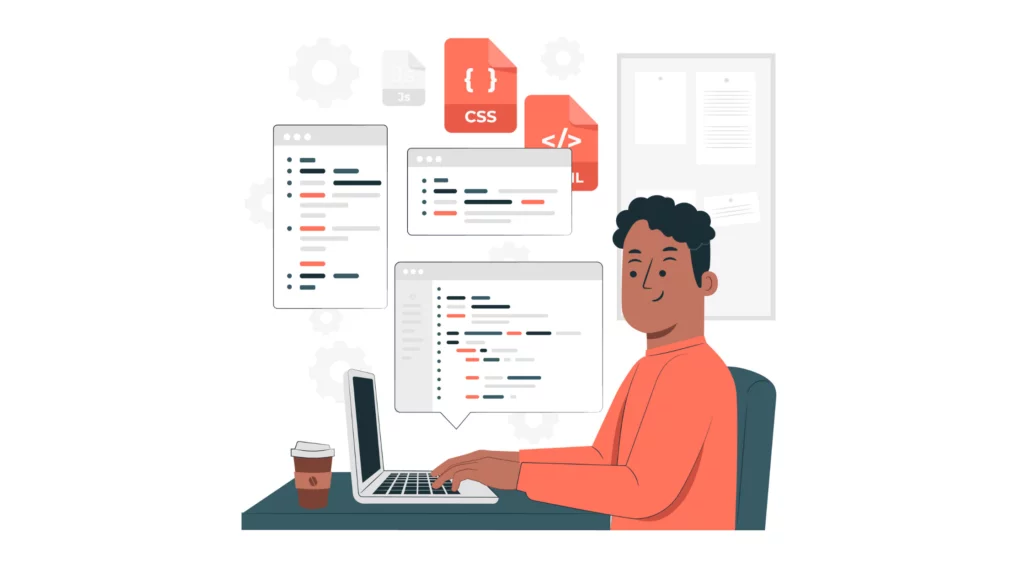
Widely popular PHP framework ‘Laravel’ has been providing a wide range of functions to facilitate and improve the world of web development. The routing mechanism of Laravel is one of its USPs. The next question that might pop into your mind is ‘what are routes in Laravel?’
In Laravel, routing is the process of connecting particular application operations to HTTP requests. According to the request’s URL, a Laravel application routes every request a user makes to a certain route. The route then chooses which closure or controller method will handle the request.
Laravel provides a straightforward and clean approach to defining routes. You can define as many routes as you need in the file routes/web.php, where the routes are defined. Let us show you how to define routes in the blog.
Routes with HTTP Requests
Information is requested and retrieved from web servers via HTTP (Hypertext Transfer Protocol) queries. While making an HTTP request, you can specify the destination URL.
For your reference, we’ve compiled a list of the most popular HTTP requests and the corresponding URL paths:
- GET request: Information is requested from a web server using this method. A URL specifying the target resource is typically part of the path for a GET request. To retrieve the homepage of a website, your request can look like this https://www.example.com/
- POST request: A web server receives your data via this request. A POST request’s path typically includes a URL that points to the script or page that will actually handle the data. To submit a form on a website, the URL could look like this: https://www.example.com/form-submit.php.
- PUT request: Updating pre-existing information on a web server necessitates a PUT request. Typically, a PUT request’s path will include a URL that points to the resource that’s supposed to be modified. If a user wants to change their contact information, one possible path is: https://www.example.com/user-profile/123
- DELETE request: If you want to remove some information from a web server, you can send a DELETE request. Typically, a DELETE request’s path will include a URL that points directly to the resource being removed. The URL https://www.example.com/user-account/123 could be used to delete a user’s account.
The above listed are just a few possible HTTP requests used to communicate with servers on the World Wide Web. The exact paths taken will vary from one online application to the next.
Read also: Laravel eCommerce or Ready-Made CMS: Which framework to Choose for Online Store?
Create routes in Laravel with HTTP requests
- In your Laravel project, open the routes/web.php file. The routes for your application will be stored in this file.
- Use the Route façade to specify a route. Use the get function, for instance, to specify a route that answers to HTTP GET requests. The following is the fundamental syntax for defining a route:
Route::get('/url', function () {
// Logic to be executed when this route is accessed
});
Change /url to the route’s URL and the anonymous function to the logic that will be carried out whenever the route is accessed.
- Refresh the page after saving the file. By going to the URL you supplied, you ought to be able to access the route right away.
By employing the corresponding methods post, put, patch, and delete, you may also construct routes for other HTTP verbs, such as POST, PUT, and DELETE.
The name method allows you to give your route a name as well. When creating URLs for named routes, this method proves beneficial. For instance:
Route::get('/users/{id}', function ($id) {
// Logic to be executed when this route is accessed
})->name('users.show');
You can generate a URL for this route using the route function as follows:
$url = route('users.show', ['id' => 1]);
This will generate a URL like /users/1.
That’s it! This is how you can create routes in Laravel.
Routing with Controller Function
Routing is often used in conjunction with controllers, which are used to group related request handling logic into a single class.
Here’s an example of defining a route that maps to a controller function in Laravel:
1. First, create a new controller using the following command in the terminal:
php artisan make:controller MyController
2. In the controller file (located at app/Http/Controllers/MyController.php), define a function that will handle the request:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class MyController extends Controller
{
public function index()
{
return view('my-view');
}
}
In this example, the index() function simply returns a view called my-view.
3. Next, define a route that maps to the index() function in the routes/web.php file:
Route::get('/my-route', [MyController::class, 'index']);
In the above example, a route that answers HTTP GET requests is defined using the get() method. The URL path that will initiate the route is the first parameter, and the second is an array with the controller and function that should handle the request.
- To test the route, go to https://your-app-url/my-route in your web browser.
Laravel will use the index() function in the MyController class to handle the request when a user accesses the URL path specified in the route. The index() function can then carry out any required processing and provide the user with a response.
Routing with Naming Function
Your routes can be given names in Laravel to make it simpler to link to them all throughout your application. Use the name method on the route specification to give a route a name.
Here’s an example of how to name a route in Laravel:
Route::get('/users', function () {
})->name('users.index');
Only through the implementation of the name method, the name users.index is assigned to the route. The route function, which generates URLs for the route, can then make use of this name elsewhere in your application.
$url = route('users.index');
Another way to use the route function is by generating URLs for named routes with parameters:
Route::get('/users/{id}', function ($id) {
// …
})->name('users.show');
$url = route('users.show', ['id' => 1]);
In the above route, a URL is created with the help of the route function user.show. where the value is set to id=1.
When you have a lot of routes in your project, naming them can help make the code more understandable and easier to manage.
Read also: How to Build a Complex Bootstrap Frontend Using Laravel Admin Panel in a Few Easy Steps
Conclusion: Create Routes In Laravel
Laravel provides a simple and straightforward method for creating routes for your online application. You can describe how your application reacts to HTTP requests for various URLs by creating routes in the routes/web.php file.
Laravel’s syntax for constructing routes is simple and easy to grasp, making it an ideal choice for both new and seasoned developers. Overall, Laravel’s route system is an effective tool for developing sturdy and efficient web applications.