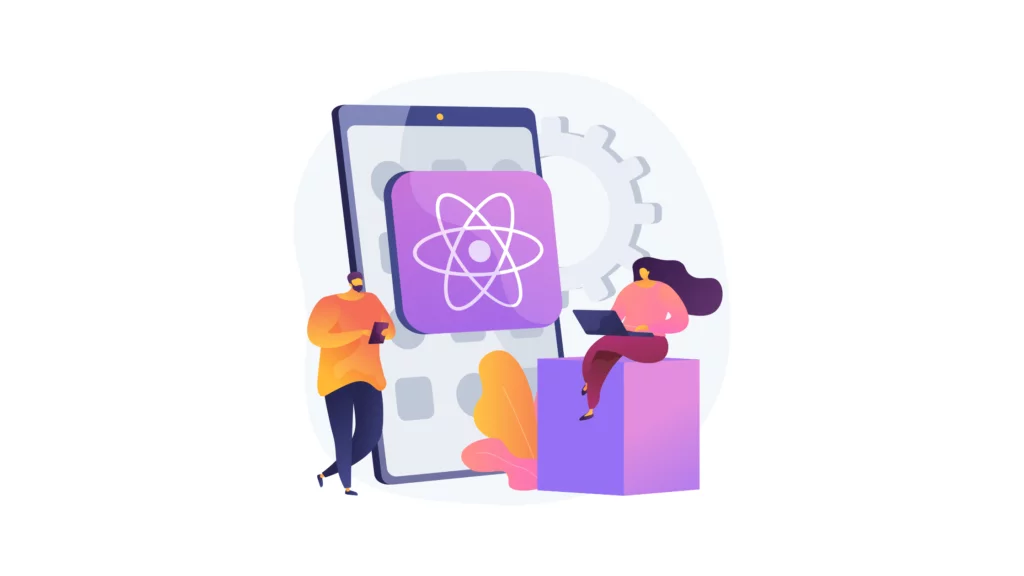
Indexability in Angular and React dramatically affects how well search engines can crawl and index certain content on a website; hence, it is a crucial component of web development. React, and Angular are two well-known JavaScript frameworks that create dynamic web applications. Indexability becomes more difficult for these two, especially with the release of Universal JavaScript.
React, and Angular are frameworks for single-page applications (SPAs) that help load a single HTML page and modify its content dynamically based on user interactions. This can add problems for search engines because content heavily relying on JavaScript for rendering might require more than just the traditional/old-school crawlers to interpret and index.
To solve this problem, developers render components on the server side before sending the HTML file to the client using Universal JavaScript, also called serverside rendering (SSR) in the context of React. As search engines can more easily index this (prerendered) content, the website’s optimization (SEO) is enhanced, and users can find relevant content using search queries.
The term “Multiple Page Application” (MPA) describes the traditional frameworks that produce all of the HTML required for spiders to properly index and navigate through the pages on the server.
On the other hand, Single Page Applications (SPAs) that, through the use of Fetch requests, generate a portion of the HTML on the client. This makes it impossible for spiders to properly index and, more importantly, track all of the content.
Additionally, these websites can be implemented without trackable links by using URLs with fragments (using the # symbol) or directly without URLs in the links (which function only for the user when they click them).
Angular (formerly AngularJS) from Google, React from Facebook, and countless other opensource frameworks like Vue, Meteor, Lazo, Render, Ember, Aurelia, Backbone, Knockout, Mercury, etc. are just a few examples of the numerous SPA-type frameworks out there. At first, these frameworks could only be executed on the client; however, as we shall see, there are better courses of action than this.
How does a SPA framework work without Universal JavaScript?
As indicated, the SPA frameworks rely on the Fetch API to function. To do this, they load into the browser along with a shell that holds the parts that remain unchanged while browsing and several HTML templates with responses to the fetch requests sent to the server.
Since the operation is different, it is necessary to distinguish between how this happens during the initial request to the website and during link navigation after it has already loaded:
A Single Page Application (SPA) framework, without Universal JavaScript or server-side rendering (SSR), primarily relies on clientside rendering for displaying content. Here’s a brief overview of how a SPA framework typically works without Universal JavaScript:
- Initial Page Load:
When a user launches the SPA for the first time, the server sends a minimal HTML document and necessary CSS and JavaScript files to the client’s browser. This HTML document is vital as it includes the main container for the application and references to JavaScript bundles.
- JavaScript Execution:
The client’s browser runs the JavaScript code, launching the SPA framework (such as Angular, React, or Vue). After that, the framework handles routing and starts to render components dynamically.
- ClientSide Routing:
The SPA framework handles clientside routing as the user interacts with the application (e.g., clicking on links or buttons). Instead of requesting new pages from the server, the SPA updates the content dynamically by manipulating the DOM.
- API Calls:
SPAs often make asynchronous API calls to fetch data from a server, typically in JSON format.
The retrieved data is then used to update the content of the application without requiring a full page reload.
- State Management:
SPAs maintain a clientside state to keep track of the current application state.
State changes trigger the rerendering of components, updating the user interface accordingly.
- Virtual DOM (React Specific):
In the case of React, a virtual DOM is used to update the actual DOM efficiently. React calculates the minimal changes needed to update the UI and applies those changes to the DOM.
Challenges That You Might Face Without Universal JavaScript: Indexability in Angular and React
SEO Implications:
Search engines may face difficulties indexing dynamic content generated using clientside JavaScript.
Traditional web crawlers might need more than asynchronous JavaScript to execute fully, potentially leading to incomplete indexing.
Initial Page Load Time:
The initial page load might be slower because the client must download and execute JavaScript before rendering the content. Users with slower internet connections or less advanced devices may experience delays.
Limited Accessibility for Bots:
Some web crawlers or bots may not execute JavaScript, potentially leading to issues with content indexing and accessibility. In contrast, Universal JavaScript or serverside rendering addresses these challenges by prerendering content on the server side, providing a more SEO-friendly and faster initial loading experience for users and search engines alike.
How did you try to make a SPA framework indexable before Universal JavaScript?
Before the widespread adoption of Universal JavaScript or server-side rendering (SSR) in single-page application (SPA) frameworks, developers often implemented various techniques to make their SPAs more indexable. Here are some common strategies:
- Prerendering:
Developers could use prerendering tools or services to generate static HTML versions of their SPA pages.
These static HTML files could be served to search engine crawlers, ensuring that search engines could index the content without relying on client-side JavaScript execution.
- Hashbang (#!) URLs:
Some developers used hashbang URLs (e.g., `example.com/#!/page`) to indicate different states of the application.
The hashbang URLs were designed to be interpreted by search engines as traditional URLs, allowing crawlers to index different “pages” within the SPA.
- Fragment Identifier (Hash) Routing:
SPA frameworks often support client-side routing using the URL’s fragment identifier (hash).
Developers could manually create routes and update the URL fragment as users navigate the application. This approach allowed search engines to see different “pages” within the SPA.
- Sitemap Submission:
Developers would manually create and maintain a sitemap.xml file containing a list of URLs inside the SPA.
This sitemap file could be submitted to search engines, providing additional information about the SPA structure and helping crawlers discover and index content.
- Dynamic Content Loading:
Some developers implemented techniques to fetch essential content during the server-side rendering phase and embed it directly into the initial HTML response.
This helped ensure crucial content was present in the initial page load, improving indexability.
- Isomorphic JavaScript:
Isomorphic JavaScript is writing code that can run on the server and the client.
Developers would leverage isomorphic libraries to share code between the server and client, allowing them to render components on the server side when necessary.
It’s important to note that while these strategies could improve indexability to some extent, they often involved additional complexities and were not as seamless as the server-side rendering provided by Universal JavaScript solutions.
With the introduction of Universal JavaScript in frameworks like Angular Universal and server-side rendering in React with Next.js, developers gained more standardized and efficient approaches to address the challenges of SPA indexability. These solutions improved SEO, enhanced initial page load times, and provided a better user experience.
Read Also: 9 Best React Native Companies For App Development In 2023
How do you make a SPA framework indexable with Universal JavaScript?
The concept of Universal JavaScript, or isomorphic JavaScript as it was initially named, is based on Facebook’s React SPA framework. It involves using a Universal API that, depending on whether it runs on the client or the server, uses client browser JavaScript APIs or server JavaScript APIs with Node.js
In this manner, we can run JavaScript code on the client and the server when writing it with this API. We have a universal framework that can operate on both the client and the server if we combine this with an SPA framework designed to run exclusively on the client:
First of all, we need to consider that, depending on where our web development is going to be executed, we can differentiate between three different types of JavaScript code:
- Only with the client.
- Only on the server, though any server language, like PHP, could take the place of this JavaScript.
- On the server as well as the client (Universal JavaScript).
We are said to be using a Full-Stack framework if we use JavaScript in the block of code that only runs on the server because we will use this language in all three scenarios.
The behavior for the first and subsequent requests will be different, just as it was when we didn’t have Universal JavaScript:
First request: the entire HTML will be generated on the server using the Universal JavaScript block, which initiates Fetch requests to the JavaScript that only runs on the server, regardless of whether a user or a spider makes the request. Except for the fetch requests being made from the server to itself rather than from the client, which saves the initial request transfer over the network, the operation is the same as when Universal JavaScript is not used.
Loading of the subsequent pages following the initial request: if a user clicks a link, the client-side JavaScript will intercept the click and forward the request to the server-side Universal JavaScript (as in the preceding point). With the requested URL, it will send a Fetch request to the JavaScript limited to the server. Once the data is retrieved, it will show the user the updated page.
In this manner, we have pages that load quickly upon a first visit and during subsequent navigation, and spiders have no trouble indexing them because the entire page is always generated on the server for these pages, negating the need for cloaking.
It is common for development companies to use an older version of the framework that does not have Universal JavaScript, so if they offer you a website built with Angular, React, or another SPA development framework, be sure they are familiar with it and have a project that is correctly indexing. Alternatively, they may need to be made aware that Universal JavaScript exists.
Read Also: What Is Indexing In SEO? Difference Between Crawling And Indexing In Search Engine
Conclusion: Indexability in Angular and React
It is common for development companies to use an older version of the framework that does not have Universal JavaScript, so if they offer you a website built with Angular, React, or another SPA development framework, be sure they are familiar with it and have a project that is correctly indexing.
Alternatively, they may need to be made aware that Universal JavaScript exists.
I hope you found this simple guide on Indexability in Angular and React with Universal Javascript helpful. Please share it with your friends if you know they might be interested in it or looking for something similar.
You Might Like: 5 Best Code Optimization Plugins For WordPress In 2024
FAQs: Indexability in Angular and React
What is Universal JavaScript in the context of Indexability In Angular and React?
Universal JavaScript refers to the ability of a JavaScript application to run on both the server and the client. In the context of Angular and React, it involves server-side rendering (SSR) to improve indexability by search engines.
Why is indexability in Angular and React so important?
Search engines traditionally struggle with indexing content rendered on the client side. Indexability ensures that search engines can properly crawl and index the content of Angular and React applications, improving SEO and discoverability.
How does Universal JavaScript address indexability in Angular and React?
Universal JavaScript enables server-side rendering, allowing the initial HTML to be generated at the server and sent to the client. This helps search engines index the content, as they can process the HTML without relying solely on client-side rendering.
Which libraries or frameworks facilitate Universal JavaScript in Angular?
For Angular, libraries like Angular Universal provide support for server-side rendering. It allows developers to create Angular applications that can be rendered on the server, improving indexability.
In React, what tools or frameworks support Universal JavaScript and server-side rendering?
React developers often use Next.js or Razzle to implement server-side rendering. These frameworks simplify the process of building React applications rendered on the server, enhancing indexability.