Laravel is a world-famous PHP framework used for building web applications. One of the key features of Laravel is its support for the Model-View-Controller (MVC) architecture, which helps to organize code and separate concerns.
Laravel Controllers are responsible for handling HTTP requests and returning responses. In the MVC architecture, Controllers sit between Models and Views and are responsible for retrieving data from the Model and passing it to the View. To create a Controller in Laravel, you can use the make:controller Artisan command or manually create a PHP file in the app/Http/Controllers directory.
In the Controller, you can define methods to handle different HTTP requests. For example, you can define a method to handle a GET request to retrieve all users from the database. Inside the method, you can retrieve the users from the User Model and then pass them to the Users View using the view() function. The Users View can then render the users using simple PHP syntax.
In this blog, we’ll take a closer look at Models and Views in Laravel and how they work together to build web applications.
What are Models in Laravel?
A Model in Laravel is a PHP class that symbolizes a database table. It is in charge of maintaining the data in the table and giving users a way to interact with it. Records in the database can be retrieved, added to, updated, and deleted using models.
Consider models to be the stewards of your data. They guarantee the validity, accuracy, and safety of the data. For instance, you would develop a User Model in Laravel if your database contained a user table. The User Model would be in charge of getting user records out of the database, adding new records for users, editing already existing records, and deleting records.
Models are implemented as extensions to the Eloquent ORM (Object-Relational Mapping) architecture in the form of PHP classes. By enabling developers to interact with database tables as though they were objects, Eloquent offers a straightforward and elegant approach to working with databases. You can manually create a PHP file in the app/Models directory or use the make:model Artisan command to create a Model in Laravel.
In order to perform CRUD (establish, Read, Update, Delete) activities on database records, you must first establish a Model. Use the all() method on the Model, for instance, to get every record from a table. You can build a new instance of the Model and then call the save() method to produce a new record. An existing record can be updated by retrieving it with the find() function, changing its attributes, and then executing save(). You can use the search() method to find a record that you want to delete, then call the delete() method.
Create
Use the create() method on your Model to add a new record to your database table. Here’s an illustration:
public function store(Request $request)
{
$product = new Product;
$product->name = $request->input('name');
$product->description = $request->input('description');
$product->price = $request->input('price');
$product->save();
return redirect()->route('products.index');
)
To save the new record to the database, first construct a new instance of the Product Model class, set its attributes using the information from the request, and then execute the save() method.
Read
Find() function can be used to locate a specific record by its ID or the all() method to retrieve all records from your database table. Here’s an illustration:
public function index()
(
= Sproducts Product::all();
return view('products.index', [ 'products' => $products]);
public function show($id)
(
product Product::find($id);
return view('products.show', ['product' => $product]
):
}
In this illustration, fetch all Product records using the all() function and give them to the index View. A different approach that you can take is by fetching a single Product record using the find() function and offering it to the show View.
Update
You can use the find() method to obtain a record from your database table, change the record’s attributes, and then call save() to save the changes. Here’s an illustration:
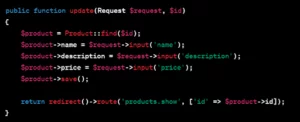
In this example, you can use the find() function to get the Product record by its ID, alter the attributes using the information from the request, and then employ the save() method to update the record in the database.
Delete
Use the delete() function on your Model to delete a record from your database table. Here’s an illustration:
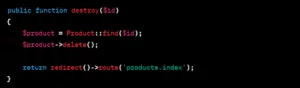
In this example, you can use the find() method to uncover the Product record by its ID and then execute the delete() method to remove it from the database.
Saving Data in Models Using Eloquent
To save data using Eloquent, you will have to primarily create a Model that corresponds to the table in your database that you want to save data to. The Model should extend the Illuminate\Database\Eloquent\Model class and define a $fillable or $guarded property to specify which attributes are mass assignable. Mass assignment is the process of setting multiple attributes of a Model at once.
Here’s an example of a simple User Model:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $fillable = [
'name'
'email'
'password',
];
}
In this example, the User Model has three attributes that are mass assignable: name, email, and password.
Eloquent authorizes you to construct a new instance of the Model and then call the save() method to add a new entry to the database. Here’s an illustration:
$user = new User;
$user->name = 'John Doe';
$user->email = 'john.doe@example.com';"
$user->password = bcrypt('secret');
$user->save();
In this demonstration, a fresh instance of the User Model is created, and its attributes are set using the dot notation. Before the password is saved to the database, it is hashed using the bcrypt() function. In order to save the record to the database, we call the save() method at the end.
Using Eloquent, you can fetch an existing record using the find() function, modify its attributes, and then use save(). Here’s an illustration:
The find() method is used in this example to fetch the User record with ID 1, which is then modified to change its name attribute. To update the database record, you can call the save() method at the completion.
Eloquent makes data saving in Laravel a quick and easy process. You may easily save and update data in your database by utilizing Models and basic PHP syntax.
What are Views in Laravel?
In Laravel, a View is a PHP file that contains HTML and PHP code. Views are responsible for rendering the user interface of your web application. They provide a way to display data to the user and allow the user to interact with the application.
Think of Views as the front-end of your application. They determine how your application looks and feels to the user. For example, if you have a page that displays a list of users, you could create a Users View in Laravel. The Users View would contain HTML and PHP code that displays the list of users retrieved from the User Model.
Views in Laravel are implemented as Blade templates. Blade is a templating engine that provides a simple syntax for creating reusable templates. Blade templates are most prominently stored in the resources/views directory and also have a .blade.php file extension.
The Laravel view() function can be used to render a View. It can be performed by accepting the View’s name as its first parameter and an optional array of data as its second argument. The View can then use primary PHP syntax to access and analyze the data. You can retrieve an array of data with the key user that you present to the View, for instance, by using the $users variable.
public function index()
{
$users User::all(); =
return view('users.index', [
'users' => $users
]);
}
In this example, we retrieve all users from the database using the all() method on the User Model and pass them to the View with the key users.
Then, in the corresponding Blade View file (resources/views/users/index.blade.php), you can access the users array using double curly braces ({{ }}) like this:
@foreach ($users as $user)
<p>{{ $user->name }}</p>
@endforeach
In this illustration, we use a foreach loop to iterate over each user in the users array and display their name using Blade syntax ({{ $user->name }}). Blade syntax allows you to easily output data to your HTML without having to write PHP code.
In Blade, you can also use if-else and loops. Refer to the official Blade documentation to learn more about Blade function.
How do Models and Views work together?
Data that Views display to the user is provided by Models. Data is pulled from the Model and passed to the View via the Controller when a user requests a page. The HTML and PHP code is then rendered by the View and sent back to the user’s browser for display.
Conclusion
The Laravel framework is not complete without models and views. The management of database data and the provision of a user interface for interacting with it are the responsibilities of models. The user interface of your web application is rendered through views. Together, they collaborate to create a seamless user experience and make sure your application is structured and upkeep-friendly.