PHP has been one of the greatest and most widely used programming languages the development world has ever seen. Some factors that enhance the performance of the programming language while some mar the performance. One such factor is PHP memory. PHP memory limit refers to the maximum amount of memory that a PHP script is allowed to use during its execution. This limit is specified in the php.ini configuration file or can be set programmatically using the ini_set() function.
Why is there a limit on PHP memory?
The memory limit is important because PHP scripts can use a lot of memory, especially when dealing with large data sets or performing complex calculations. If a script exceeds the memory limit, it may cause errors or even crash the server.
Setting a reasonable memory limit can help prevent these issues and ensure that your PHP scripts run smoothly. However, it’s important to note that setting the limit too high can also have negative consequences, such as slowing down the server or allowing poorly optimized scripts to consume too many resources.
In general, it’s a good idea to monitor the memory usage of your PHP scripts and adjust the memory limit as needed to balance performance and stability. You can use tools like memory_get_usage() and memory_get_peak_usage() to track memory usage in your scripts.
How does the PHP memory limit affect the performance of applications?
The PHP memory limit can have a significant impact on the performance of your PHP applications. Here are a few ways in which the memory limit can affect performance:
- Script Execution Time: If your PHP script demands more memory than the PHP memory limit permits, it may terminate and may generate errors. This can lead to lengthier script execution times and have an impact on the performance of other scripts running on the server.
- Server Resource utilization: Setting the PHP memory limit too high can result in higher server resource utilization, such as CPU and disk I/O. This can cause the server to slow down and have an impact on the performance of other apps running on the server.
- Memory Allocation: If a PHP application consumes more memory than the PHP memory limit allows, the operating system may request additional memory allocation. This might be a time-consuming operation that has an impact on the application’s overall performance.
- Garbage Collection: A garbage collector is used by PHP to free up memory that is no longer being utilized by a script. If the PHP memory limit is set too low, the garbage collector may be unable to keep up with demand, resulting in performance problems.
In general, it’s important to set the PHP memory limit to an appropriate level that balances performance and stability. This will help ensure that your PHP applications run smoothly without consuming excessive server resources or causing performance issues.
How to increase the PHP memory limit?
There are a few ways to increase the PHP memory limit:
- Edit the php.ini File: You can increase the PHP memory limit by editing the php.ini configuration file. This file is typically located in the root directory of your server. Look for the memory_limit directive and increase its value. For instance, to increase the memory limit to 256MB, you can set memory_limit = 256M.
- Using ini_set() Function: You can also increase the PHP memory limit programmatically using the ini_set() function in your PHP script. Simply add the following line of code to your script before the part that requires more memory: ini_set(‘memory_limit’, ‘256M’);.
- Using .htaccess File: If you do not have access to the php.ini file or prefer to modify the PHP memory limit for a specific directory only, you can use the .htaccess file. Simply add the following line of code to your .htaccess file: php_value memory_limit 256M.
Note that there are better solutions to performance issues than increasing the PHP memory limit. It is essential to identify the root cause of the performance issue and address it appropriately. If your script requires a lot of memory, consider optimizing your code or upgrading your server resources instead of simply increasing the PHP memory limit.
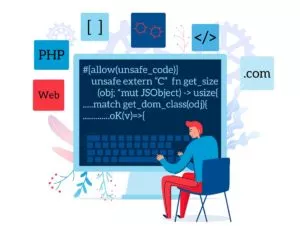
PHP memory limit for a high-traffic ecommerce site
A starting point of roughly 6 GB of memory is recommended for a high-traffic ecommerce site, however this may need to be expanded based on the site’s individual needs.
Other factors that can affect performance include optimizing database queries, caching, employing a content delivery network (CDN), and other server optimizations. Finally, monitoring the site’s performance and adjusting the memory limit as needed is the best way to discover the proper PHP memory limit for your high-traffic ecommerce site.
PHP memory limit for a high-traffic LMS site
Due to the requirement to store and process significant amounts of data, such as user information, course materials, and user progress, an LMS often requires more RAM than a standard website. As a result, for a high-traffic LMS site, a reasonable starting point for the PHP RAM limit would be roughly 512MB to 1GB, but this may need to be increased based on the site’s individual needs.
Aside from the PHP memory constraint, it is critical to optimize the server and LMS platform for performance. Caching, streamlining database queries, enabling compression, and other server improvements are examples of this. It’s also critical to keep an eye on the site’s performance and increase the PHP RAM limit as needed to keep things running smoothly and efficiently.
PHP memory limit for a high-traffic multisite
A multisite WordPress installation permits the creation of many sites from a single WordPress installation. Each site may have its own set of plugins, themes, and content, which can affect the server’s overall memory utilization.
Because of the additional database queries and memory usage associated with administering many sites, a multisite installation will often require more memory than a single site. The PHP memory limit for a high-traffic multisite would be roughly 16 GB as a starting point, but this may need to be adjusted based on the number of sites and the individual needs of each site.
It’s also critical to optimize the server and the WordPress platform for speed. Caching, streamlining database queries, enabling compression, and other server improvements are examples of this.
Conclusion
The PHP memory limit is an important part of maintaining a PHP application’s performance and stability. Increasing the PHP RAM limit can assist increase application speed, but other components of the server and application, such as caching, database queries, and server configurations, must also be optimized. It is also suggested to evaluate the site’s performance and resource utilization on a regular basis to verify that the PHP memory limit is sufficient and that it is modified as needed.
Developers may guarantee that their PHP applications function optimally and provide the greatest user experience possible by understanding the PHP memory limit and how to manage it effectively.
FAQs
What is the maximum amount of memory that PHP can use?
Depending on the setup of the server, the default PHP memory limit can be anywhere from 128MB to 2048MB.
Where can I look to see what PHP’s memory limit is?
To find out how much memory PHP can use, make a PHP file and insert this line of code:?php phpinfo();?> and get to it using a web browser. The phpinfo() result will reveal the PHP memory limit in the “Core” column.
What happens if I use more RAM than PHP allows?
If a PHP script uses too much memory, an error will be generated and the server may crash.
How do I boost PHP’s memory allocation?
The.htaccess file, the php.ini configuration file, and the ini_set() function in your PHP script all allow you to raise the PHP memory limit.
What is the optimal PHP memory limit for a busy server?
Many things go into determining the optimal PHP memory limit for a high-traffic website, including the site’s complexity and size, the amount of concurrent users, and the server configuration. For high-traffic websites, 512MB to 1GB of RAM is a good baseline.
How frequently should I check the PHP memory limit and make changes as needed?
The site’s performance and resource consumption are two factors that should guide how much RAM PHP is allowed to use. To keep your site running quickly and efficiently, you should check its performance often and increase or decrease the PHP memory limit as necessary.