Developers must ensure their apps perform efficiently, as even a one-second delay in load time can lead to a 26% drop-in conversion rates, according to Akamai research. React memoization is a crucial technique to enhance client-side performance, though it does come with a slight increase in memory usage.
Understanding Memoization:
Memoization is a programming technique where computational results are stored and linked to their inputs. This caching allows quicker retrieval when the same function is called again, making it a fundamental aspect of React’s architecture.
Memoization in React
React developers can access three memoization hooks to optimize different parts of their applications. Let’s delve into memoization, the types of React hooks, and their appropriate usage.
Memoization is a long-standing optimization technique used at the function level in software and instruction level in hardware. While it benefits repetitive function calls, it has limitations due to its memory usage. Hence, memoization should be applied to functions with expensive computations rather than cheap functions called frequently with varied arguments. Additionally, memoization is suited for pure functions, which are deterministic and have no side effects.
A General Algorithm for Memoization
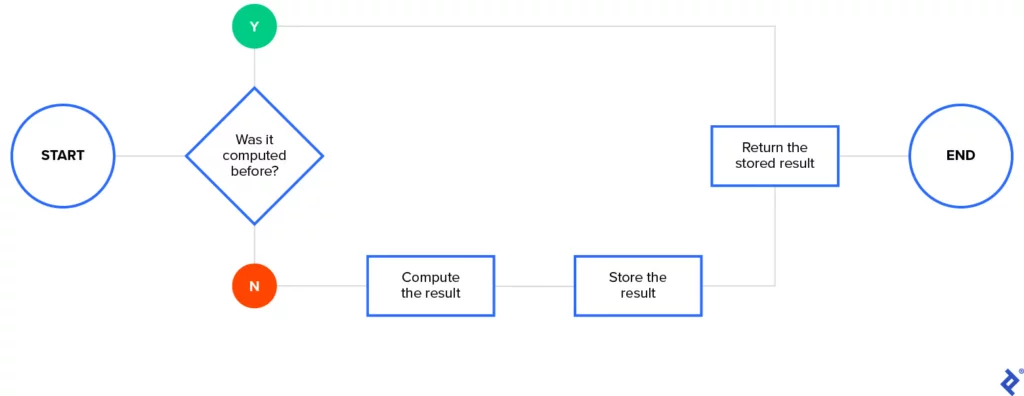
A simple flowchart outlines the memoization logic in React. It begins with a decision node checking if the result was previously computed. If yes, the stored result is returned. Otherwise, the result is computed, stored, and then returned. Both paths lead to a common “Return the stored result” node, concluding the process.
Memoization necessitates at least one cache, typically a JavaScript object in JavaScript. Other languages use similar key-value pair implementations. To memoize a function, create a cache object and add results as key-value pairs.
Each function’s unique parameter set defines a key in the cache. The function’s result is stored with that key. For functions with multiple input parameters, concatenate the arguments with dashes to form the key. This method allows quick reference to cached values.
Applying Memoization in React
Memoization in React is applied through specific hooks, depending on the optimization needs of the application. Understanding when and how to use these hooks can significantly improve app performance.
By leveraging memoization effectively, React developers can create apps that load faster and provide a seamless user experience, crucial for maintaining high conversion rates and user satisfaction.
Functions for Memoization in React:
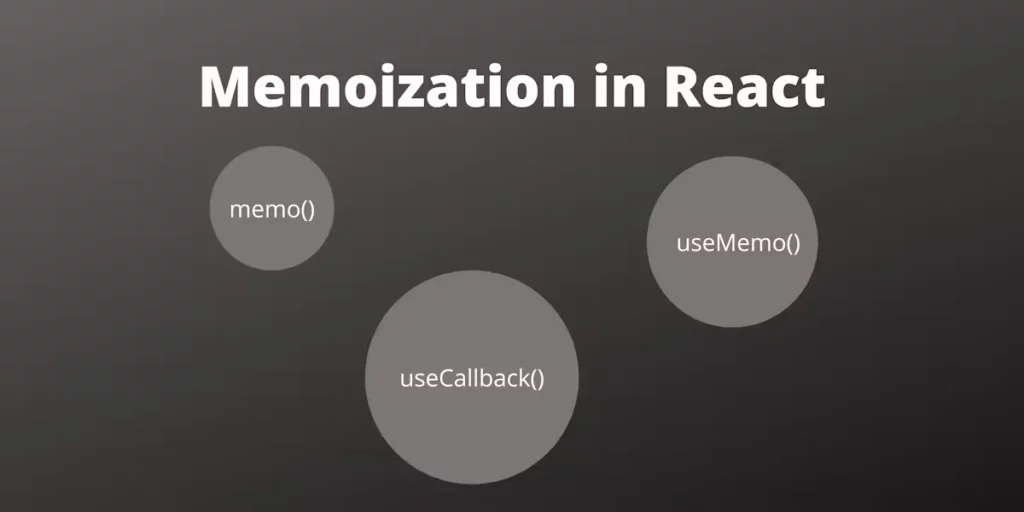
React applications typically feature highly responsive user interfaces with swift rendering. However, as applications scale, developers may encounter performance issues. Memoization in React can help mitigate these concerns by speeding up component rerenders. There are three primary React memoization functions and hooks: memo, useCallback, and useMemo.
React.memo
To memoize a pure component, wrap it with React.memo. This function memoizes the component based on the props, meaning React saves the component’s DOM tree in memory and returns this saved result instead of rerendering the component with the same props.
It’s important to note that React memo makes a shallow comparison between previous and current props. This might not trigger the memoized result retrieval if dependencies outside these props are involved. React.memo is most effective when a parent component’s updates cause unnecessary rerenders of child components.
import React, { useState } from 'react';
{user.name}
// Memoized User component
const User = React.memo(({ user }) => (
{user.address}
));
const NameInput = ({ name, handleNameChange }) => (
handleNameChange(e.target.value)}
placeholder="Search by name"
/>
);
const App = () => {
const [name, setName] = useState('');
const users = [
{ name: 'Angie Beard', address: '255 Bridge Street, Buxton, Maryland, 689' },
{ name: 'Jeannette Horn', address: '553 Glen Street, Kramer, Wisconsin, 6556' },
// …more users
];
const filteredUsers = users.filter(user => user.name.includes(name));
return (
{filteredUsers.map(user => )}
);
};
export default App;
React.useCallback
In React development, component memoization relies on props remaining unchanged. However, JavaScript function references often change between renders, potentially breaking memoization when these functions are passed as props to child components. The React.useCallback hook addresses this issue by ensuring function props remain consistent.
Using useCallback is particularly beneficial when passing callback functions to components that are either medium or high in complexity and we want to prevent unnecessary rerenders.
Example:
Let’s enhance our previous example. We add functionality so that clicking a User component updates the filter field with that user’s name. To do this, we pass the handleNameChange function to the User component, which executes the function when a user is clicked.
Components:
- NameInput: A functional component that takes an input state and an update function (handleNameChange).
- User: A memoized component that renders user details and triggers the handleNameChange function on click.
- App: The main component containing the overall logic, including the memoized handleNameChange function using useCallback.
import React, { useState, useCallback } from 'react';
// Memoized User component
const User = React.memo(({ user, onClick }) => (
onClick(user.name)}>
{user.name}
{user.address}
));
const NameInput = ({ name, handleNameChange }) => (
handleNameChange(e.target.value)}
placeholder="Search by name"
/>
);
const App = () => {
const [name, setName] = useState('');
const users = [
{ name: 'Angie Beard', address: '255 Bridge Street, Buxton, Maryland, 689' },
{ name: 'Jeannette Horn', address: '553 Glen Street, Kramer, Wisconsin, 6556' },
// …more users
];
// Memoize handleNameChange using useCallback
const handleNameChange = useCallback((newName) => {
setName(newName);
}, []);
const filteredUsers = users.filter(user => user.name.includes(name));
return (
{filteredUsers.map(user => ( ))}
);
};
export default App;
By using useCallback, the handleNameChange function retains its reference across renders, preventing unnecessary rerenders of the User component and enhancing overall performance. This approach ensures memoization remains effective even when functions are passed as props.
React.useMemo
In React, we can leverage memoization to optimize expensive operations and computations within a component using the useMemo hook. This hook is ideal for running calculations that depend on specific variables, referred to as dependencies. The useMemo hook takes two arguments:
- The function that calculates and returns a value.
- The dependency array is required to calculate that value.
useMemo calls the provided function to compute a result only when any of the listed dependencies change. React will use the memoized return value if the dependency values remain unchanged instead of recomputing the function.
Example:
Let’s modify our previous example to perform an expensive user array calculation. We will calculate a hash for each user’s address before displaying it:
Explanation:
- NameInput: A functional component that takes an input state and an update function (handleNameChange).
- User: A memoized component that renders user details and triggers the handleNameChange function on click.
- App: The main component containing the overall logic, including the memoized handleNameChange function using useCallback and the memoized calculation of hashed addresses using useMemo.
In this example, the expensive computation of hashing each user’s address is now wrapped in the useMemo hook. This ensures that the computation only occurs when the users array changes, avoiding unnecessary recalculations on every render caused by updates to the filter field.
import React, { useState, useCallback, useMemo } from 'react';
import sha512 from 'crypto-js/sha512';
import NameInput from './components/NameInput';
import User from './components/User';
import users from './data/users';
function App() {
const [name, setName] = useState('');
const handleNameChange = useCallback((name) => setName(name), []);
// Memoize the calculation of hashed addresses
const newUsers = useMemo(
() =>
users.map((user) => ({
…user,
address: sha512(user.address).toString(),
})),
[users]
);
return (
{newUsers.map((user) => ( ))}
);
}
export default App;
The Impact of Page Weight on Website Performance: Best Practices for Optimization in 2024
Should We Memoize Everything in React?
Memoization is a powerful optimization technique in React, but it’s not without its costs. Before memoizing every component, it’s important to understand the implications and trade-offs involved. Here are the three main costs associated with adding memoization to an application:
- Increased Memory Usage:
- Memory Overhead: Memoization increases memory usage because React needs to store memoized components and values. Each memoized component’s previous render is saved for comparison with subsequent renders.
- Management Challenges: If too many components are memoized, the application may need help managing its memory usage efficiently. Although the memory overhead of React.memo is minimal due to shallow comparisons, it can add up in large applications.
- Example: Companies like Coinbase memoize every component because the cost is relatively low. However, this approach may only be suitable for some applications, especially those with limited resources.
- Computation Overhead:
- Comparison Costs: When memoizing, React compares previous values to current values to determine if a component should re-render. This comparison process itself adds computational overhead.
- Cost-Benefit Analysis: Typically, the overhead from these comparisons is less than the cost of additional renders or computations. However, memoization might cost more in terms of performance for very small components with numerous comparisons than it saves.
- Example: Memoizing a simple button component that renders frequently might not provide significant performance benefits and could lead to unnecessary computation overhead.
- Increased Code Complexity:
- Boilerplate Code: Adding memoization hooks such as React.memo, useCallback, and useMemo increases the amount of boilerplate code. This can make the codebase more complex and harder to read.
- Readability vs. Performance: Developers often must balance performance improvements with code readability. While optimizing for performance is crucial, ensuring the code remains maintainable and understandable is also important.
- Example: In a large application, excessive memoization hooks might make it easier for new developers to understand the code, leading to potential maintenance challenges.
How to Hide Your API Key in React Application (Safest Way 2024)
When to Use Memoization:
Memoization should be used strategically, typically during the optimization phase of application development. Here are some guidelines:
- Optimize Critical Paths: Focus on memoizing components that significantly impact performance, such as those involved in frequent renders or expensive computations.
- Profile Before Optimizing: Use performance profiling tools to identify bottlenecks in your application. Only add memoization where it provides a clear benefit.
- Avoid Premature Optimization: Resist the urge to memoize every component. Instead, write clean and functional code first, and optimize later based on actual performance data.
Indexability in Angular and React with Universal JavaScript: Latest 2024 Edition
Summary:
Memoization in React is a valuable tool for improving application performance but comes with trade-offs. The main costs to consider are increased memory usage, computation overhead, and code complexity. By understanding these costs and applying memoization judiciously, developers can ensure their applications remain performant without compromising maintainability. The key is to use memoization strategically, focusing on the most impactful areas and balancing optimization with code readability.
FAQs on Memoization:
Can memoization increase memory usage?
Yes, memoization increases memory usage because React stores memoized components and values. While this overhead is generally minimal, excessive memoization can lead to significant memory usage.
How can I determine which components to memoize?
Use performance profiling tools to identify components that are causing performance bottlenecks. Memoize components involved in frequent renders or expensive computations to achieve the most significant performance gains.