Many developers utilize the well-liked PHP framework Laravel to create online applications. Laravel’s caching system, which enables you to save frequently accessed data on disk or in memory and enhance the performance of your application, is one of its core features.
Caching is crucial because it can increase an application’s performance by minimizing the amount of work needed to generate or retrieve data. Data that is often requested is cached so that it can be quickly retrieved without needing to be recalculated or obtained from its original source. Laravel caching can be done in memory or on storage.
Laravel Caching
A straightforward and adaptable caching API is offered by Laravel and supports a number of caching drivers, including file, database, Redis, and memcached. The caching API offers functions like cache tags, expiration times, and automatic cache validation and enables you to save, retrieve, and invalidate cached data. Use Laravel caching in your application in the following ways:
- Database query Laravel caching: Laravel caching can be used to save the outcomes of frequently used database queries. Because fewer database queries will need to be made, your application’s performance will be greatly enhanced. You can use the remember method of the query builder to cache a database query:
$users = DB::table('users')->where('name', 'John')->remember(10)->get();
This will cache the results of the query for 10 minutes.
- Caching views: Viewing of output can be majorly and conveniently cached using Laravel caching. By minimizing the time necessary to render views, you can greatly enhance the performance of your application. You can cache a view by using the cache method in your view:
{{ cache()->remember('my_view', 10, function () {
return view('my_view');
}) }}
This will cache the output of the view for 10 minutes.
- Caching API responses: Laravel caching can be a great feature to be used to cache API request responses. This can dramatically increase your application’s performance by minimizing the time necessary to retrieve data from external APIs. To cache an API response, employ the HTTP client’s remember method:
$response = Http::withHeaders([...])->get('https://api.example.com')->remember(10);
This will cache the response for 10 minutes.
- Caching application data: Laravel caching can be used to cache application data such as configuration files, translations, and other frequently used data. You can put to use the Cache façade to cache application data:
$value = Cache::remember('my_key', 10, function () {
// Code to generate the value
});
This will cache the value for 10 minutes.
Laravel caching is a powerful and adaptable tool that can dramatically boost the performance of your application. Caching frequently used data allows you to reduce the time it takes to generate or retrieve data, resulting in faster load times, a better user experience, and cost savings.
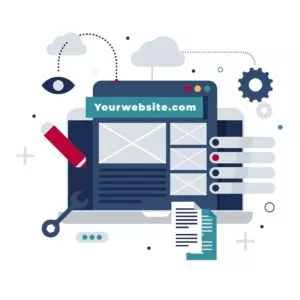
Steps to Integrate Laravel Caching into your Application
Step 1: Configure Laravel Caching
You must set up the Laravel caching in your application before you can begin using it. Change the default cache driver to the one you want to use by opening the config/cache.php file. Different cache drivers, including file, database, redis, and memcached, are supported by Laravel. For instance, edit the configuration as follows if you want to use the file driver:
'default' => env('CACHE_DRIVER', 'file'),
Other parameters, such as the cache prefix, the time-to-live (TTL) of cached items, and the cache store you want to utilize, can also be customized.
Step 2: Store Data in Cache
You can begin storing data in the Laravel cache once it has been configured. Utilizing the Cache facade, which offers a straightforward API for adding to and removing data from the cache, is the easiest approach to store data. For instance, you may use the following code to keep a value in the cache for 10 minutes:
use Illuminate\Support\Facades\Cache;
Cache::put('key', 'value', 10);
This will store the value ‘value’ under the key ‘key’ in the cache for 10 minutes. Using the get method, you may receive the value later:
$value = Cache::get('key');
The get method will return null if the value is either not in the cache or has expired.
Step 3: Cache Tags
One of the powerful features of Laravel cache is the ability to tag cached items. Tags allow you to group related items together and perform operations on them, such as invalidating all items with a particular tag. To tag a cached item, use the tags method:
Cache::tags(['tag1', 'tag2'])->put('key', 'value', 10);
This will store the value under the key ‘key’ with the tags tag1 and tag2. You can retrieve the value using the get method as before, but you can also invalidate all items with a particular tag using the forget method:
Cache::tags('tag1')->forget('key');
This will remove the item with the key ‘key’ and the tag ‘tag1’ from the cache.
Step 4: Cache Drivers
As mentioned earlier, Laravel supports various cache drivers. Each driver has its own advantages and disadvantages, and you should choose the one that best suits your needs. Here is a brief overview of the available drivers:
File: stores cached items in files on disk.
Database: stores cached items in a database table.
Redis: stores cached items in a Redis database.
Memcached: stores cached items in a Memcached server.
To use a specific driver, update the CACHE_DRIVER environment variable in your .env file:
CACHE_DRIVER=redis
This will use the Redis cache driver.
Step 5: Laravel Caching Validation
Finally, cached data must be authenticated to ensure its validity and updated to current date. If a value does not already exist in the cache or has expired, you can use the remember method for storing it:
$value = Cache::remember('key', 10, function () {
// Code to generate the value
});
If the value does not already exist or has expired, it will be saved for 10 minutes. If the value is already in the cache, it will be returned instantaneously.
Software used to test Laravel Caching
There are various software tools that can be used to test the Laravel cache. Here are a few options:
PHPUnit is the standard testing framework for PHP applications and is included with Laravel. It allows you to write unit tests to test your Laravel application’s functionality, including cache operations.
Mockery is a library for creating mock objects in PHP that can be used in unit tests to isolate and test specific parts of your code. It can be used in combination with PHPUnit to test Laravel cache operations.
Laravel Dusk is a browser automation and testing tool for Laravel applications. It can be used to test the behavior of your application from a user’s perspective, including cache operations.
Laravel Tinker is a command-line interface for interacting with your Laravel application’s code. It can be used to test cache operations by allowing you to manually manipulate the cache store and test its behavior.
Blackfire.io is a performance profiling tool that can test the performance of your Laravel application, including its cache operations. It can help you identify performance bottlenecks and optimize your application’s cache usage.
By using these software tools to test Laravel cache, you can ensure that your application’s caching behavior is working as expected and optimize its performance.
Using Laravel Caching for Testing
You might want to verify that the caching behavior behaves as intended while testing an application that makes use of Laravel Cache. By offering a testing cache driver, Laravel offers an easy and effective approach for you to test caching in your application.
The steps to test using Laravel Cache are as follows:
Cache driver should be set to “array”
Set the cache driver to ‘array’ for your testing environment in the config/cache.php file. This will guarantee that every cache operation is carried out in memory and does not last across test runs.
return [
// ...
'default' => env('CACHE_DRIVER', 'file'),
// ...
'stores' => [
// ...
'array' => [
'driver' => 'array',
],
// ...
],
// ...
];
Test caching data and retrieving it
You may test storing and retrieving cached data as follows after defining the testing cache driver in your test case using the Cache::store(‘array’) method:
use Illuminate\Support\Facades\Cache;
use Tests\TestCase;
class CacheTest extends TestCase
{
public function testCache()
{
// Store a value in the cache
Cache::store('array')->put('my_key', 'my_value', 10);
// Retrieve the value from the cache
$value = Cache::store('array')->get('my_key');
// Assert that the value is correct
$this->assertEquals('my_value', $value);
}
}
Test erasing data from cache
Using the Cache::store(‘array’) method to provide the testing cache driver and testing the forget or flush methods as follows will allow you to test invalidating cached data as well:
use Illuminate\Support\Facades\Cache;
use Tests\TestCase;
class CacheTest extends TestCase
{
public function testCache()
{
// Store a value in the cache
Cache::store('array')->put('my_key', 'my_value', 10);
// Remove the value from the cache
Cache::store('array')->forget('my_key');
// Assert that the value is null
$this->assertNull(Cache::store('array')->get('my_key'));
// Store another value in the cache
Cache::store('array')->put('my_key2', 'my_value2', 10);
// Remove all values from the cache
Cache::store('array')->flush();
// Assert that both values are null
$this->assertNull(Cache::store('array')->get('my_key'));
$this->assertNull(Cache::store('array')->get('my_key2'));
}
}
You can make sure that your application’s caching behavior is functioning as intended and lower the likelihood of unexpected behavior in production by utilizing the testing cache driver and testing caching behavior.
Conclusion
Laravel caching is a crucial method for enhancing the efficiency and scalability of applications. Caching can enhance an application’s speed, user experience, and cost-effectiveness by lowering the amount of labor necessary to generate or retrieve data.
FAQs
How does the Laravel caching operate?
The Laravel caching operates by saving data for easy access in a cache store, such as Redis, Memcached, or the file system. The application first examines the cache store when it is requested to see the data. Data is returned without having to be fetched from the original source if it already exists in the cache.
How advantageous is it to use Laravel caching method?
By cutting back the amount of databases or API calls necessary to retrieve data, using Laravel caching can reinforce the efficiency of your application. Additionally, it can help to lessen server load and increase scalability.
In Laravel, how do I create a cache store?
In your config/cache.php file, you must set up the cache driver and connection settings in order to set up a cache store in Laravel. Cache drivers used by Laravel include Redis, Memcached, and the file system.