CodeIgniter is a popular PHP framework used for web development. One of the key features of CodeIgniter is its built-in pagination library, which makes it easy to display large amounts of data in a user-friendly manner. In this blog post, we will educate you on how to use CodeIgniter pagination in your projects on any PHP hosting.
Importance of Pagination in PHP
Pagination is an essential feature in web development, including PHP, that allows you to divide large data sets into smaller, more manageable chunks. It enables users to navigate the data systematically, improving user experience and overall performance. Here are some significant reasons highlighting the importance of pagination in PHP:
- Improved Performance: When dealing with large datasets, fetching and displaying all the records simultaneously can lead to slower page load times and increased server load. Pagination helps mitigate this issue by retrieving and showcasing a limited number of records per page, reducing the processing and rendering time.
- Enhanced User Experience: Users typically prefer a streamlined browsing experience. Pagination allows users to navigate through different pages of data, making it easier to find specific information or locate desired results. It enhances usability by breaking down content into manageable portions and providing clear navigation controls.
- Reduced Bandwidth Usage: Transmitting large volumes of data over the network can consume significant bandwidth. By implementing pagination, you only retrieve a subset of data for each page, reducing the amount of information transferred between the server and the user’s browser. This optimization helps conserve bandwidth and improves overall website performance.
- Scalability: Pagination plays a crucial role in handling large datasets and scalable web applications. Without pagination, as the amount of data grows, fetching and processing all records can become increasingly burdensome for the server and impact performance. With pagination, you can easily accommodate growing data without overloading system resources.
- Search Engine Optimization (SEO): Search engines often favor websites that provide good user experiences. By implementing pagination, you create a logical structure for your content, making it easier for search engine crawlers to index and understand your website. This can positively impact your search engine rankings and organic traffic.
In PHP, you can utilize various techniques and libraries to implement pagination effectively. Common approaches involve using SQL queries with LIMIT and OFFSET clauses for database-driven applications or array slicing for in-memory data. Additionally, frameworks like Laravel and CodeIgniter provide built-in support for pagination, simplifying the implementation process.
Remember to consider factors such as appropriate page size, clear navigation controls, and maintaining context when implementing pagination in PHP.
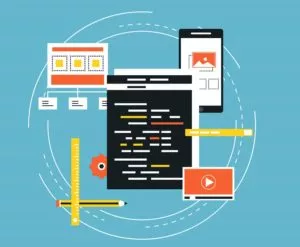
Steps to Implement Pagination in Codeigniter Projects
Step 1: Load the Pagination Library
We must initially load the pagination library into our controller in order to use it in our CodeIgniter project. To accomplish this, you must add the following piece of code to the constructor method of our controller:
$this->load->library('pagination');
This will load the pagination library and make it available for use in our controller.
Step 2: Set Pagination Configuration
Next, we’ll configure our pagination options. Included in this are the page break parameters (starting and ending counts) and the pagination URL segment. As an example of how to set up pagination, consider the following:
$config['base_url'] = base_url('example/index');
$config['total_rows'] = $this->db->count_all('example_table');
$config['per_page'] = 10;
$config['uri_segment'] = 3;
$this->pagination->initialize($config);
The base URL for the needed for the pagination links is set in the code above to example/index, which is the method in our controller that will handle the pagination. Additionally, we changed the total amount of rows to reflect how many items there are in our database’s example_table table. We set the per_page option to 10 because we want to display ten items per page. Finally, we notify CodeIgniter that the pagination section will be the third segment in the URL by setting the uri_segment option to 3.
Step 3: Retrieve Data from the Database
We can access the information we want to display from our database now that our pagination library has been loaded and our setup has been established. Here’s an illustration of how to get the info back:
$data[‘results’] = $this->db->get(‘example_table’, $config[‘per_page’], $this->uri->segment(3))->result_array();
In the code above, we use CodeIgniter’s get() method to retrieve the data from our example_table table. We pass in the per_page option from our pagination configuration, as well as the current pagination segment using the URI ->segment() method.
Step 4: Display the Data
At last, we can use a loop to serialize the data and present it on the website. To see an example of how to present the data with a for each loop, consider this:
foreach($results as $row) {
echo $row['title'];
echo $row['description'];
}
The title and description fields are displayed for each row as we loop through each result in the $results array in the code above.
Step 5: Display Pagination Links
You must utilize the native create_links() method of CodeIgniter to display the pagination links. An illustration of how to display the pagination links is given below:
echo $this->pagination->create_links();
The create_links() method is implemented in the code above to create the pagination links and display them on the page.
Understanding the variables
$config
The pagination library’s configuration variables are all stored in the $config array. The $this->pagination->initialize() method is then called with these variables as arguments, as shown in the code above.
base_url()
The “base_url()” method returns the full URL of the controller function or class where your pagination is contained. It makes reference to a function called “index,” and a controller called “Welcome” in the provided example. It’s important to know that adopting a different routing structure allows you to change your URI.
total_rows()
The paginated result set’s total number of rows is represented by the total_rows() function. This figure typically reflects the total number of rows the database query returned.
per_page()
The per_page() method sets the quantity of items to display on each page.
create_links()
If there is no pagination to display, the create_links() function will output an empty string.
uri_segment
Although the CodeIgniter paging library normally recognizes these parameters automatically from the URL, if you have a specific URL pattern, you can specify a custom value for paging-related parameters. When visiting a page, the paging URI segment, by default, reflects the starting index. For example, if your pagination arrangement has 10 records per page, the third page’s paging URI segment would be 20.
Conclusion
Implementing pagination in your CodeIgniter projects is a simple task that can significantly improve the usability and performance of your web application. By adhering to the instructions in this blog post, you can guarantee that your users can effortlessly browse through extensive data sets, enhancing the overall user experience. It is essential to carefully determine the number of items to display per page, employ suitable SQL queries, and incorporate effective error handling to establish a robust pagination system. Armed with the knowledge acquired from this guide, you are fully prepared to implement pagination in your CodeIgniter projects effectively.
FAQs
Are there any best practices for implementing pagination?
Yes, there are some best practices to consider:
- Carefully choose the number of items per page based on the nature of your data and the expected user experience.
- Optimize your SQL queries by using proper indexing and limiting the number of retrieved rows.
- Implement error handling to handle cases where users try to access pages that don’t exist.
- Consider implementing caching techniques to further improve performance, especially if the data doesn’t change frequently.
Can I customize the appearance of pagination links in CodeIgniter?
Yes, CodeIgniter’s pagination library provides options to customize the appearance of pagination links. You can define your own CSS classes and HTML markup and even use your own view files to render the pagination links according to your project’s design requirements. Refer to CodeIgniter’s documentation for more information on customization options.
Are there any alternative pagination libraries for CodeIgniter?
Yes, apart from the built-in pagination library provided by CodeIgniter, there are alternative libraries available that offer additional features and flexibility. Some popular choices include “CI-pagination” and “Datatables.” These libraries provide more advanced pagination functionalities and may be suitable for specific project requirements.