PHP’s error logs can be managed effectively through various manual and automated logging methods. Furthermore, the open-source community has provided us with some useful third-party tools for reporting PHP errors.
The timing and method of error reporting are crucial. When in dev mode, PHP errors can be logged at your discretion. You have the option of saving errors as notifications on various channels or creating a PHP error log file, depending on your needs.
The configuration and accessibility of PHP logs are the primary topics of this tutorial. This manual also explains how logging makes debugging and keeping an eye on your PHP programs much easier.
Types of PHP Errors
PHP errors arise when there is something erroneous inside the code. They can be as complicated as calling an invalid variable or as easy as skipping a semicolon. Understanding the types of errors is essential before solving them effectively.
-
Warning Error
The warnings in PHP flag potential issues before they escalate into critical problems. Errors of type “warning” are generated when an invalid file path is used, but they do not halt program execution.
If this issue occurs, for instance, you may need to double-check the file’s name in the code or directory; the script may not have been able to locate it because of the syntax problem.
-
Notice Error
Like warning errors, notice errors do not stop code execution and are therefore considered lower severity. These mistakes can make it difficult for the system to determine whether or not an error occurred or whether it is simply the expected result of a certain piece of code. The script requires access to an undefined variable, which causes a notice error.
-
Syntax Error
Most syntax errors result from misplaced, mistyped, or misinterpreted symbols. The script is terminated when the error is detected by the compiler.
Syntax and parsing problems arise when semicolons and parentheses are missing or used incorrectly, due to misspellings, or due to open brackets or quotes.
-
Fatal Error
A fatal error occurs if the function is called in the code yet the function is undefined. A fatal error in PHP stops the program from running and may even cause it to crash. Fatal errors fall into three categories:
- When the framework is unable to run the code because of an error in the installation process, a fatal error is reported during startup.
- When a developer makes use of a variable or data type that does not exist, a fatal error is generated at compile time.
- A fatal error that occurs during program execution is called a runtime fatal error and is identical to the compile time fatal error.
PHP Error Logging
Most websites and web apps today are written in PHP. According to w3techs, PHP powers 77.55% of websites across the globe as a server-side programming language. These numbers pinpoint that PHP continues to have a sizable part of the programming language industry.
When you first begin developing a PHP program, you use commands like print r() and var dump() to debug the errors and log in to the site. Working in production mode, however, requires a more secure method. You can do that in the development mode, but you must disable it while migrating.
Thus, when in development mode, logging errors in PHP is as simple as calling the error log() function, which will then forward the error message to the program’s predefined error handling procedures. If, for example, PHP is unable to establish a connection to a MySQL database, you can log key errors in the following manner:
<?php
// Send error message to the server log if error connecting to the database
if (!mysqli_connect("localhost","bad_user","bad_password","my_db")) {
error_log("Failed to connect to database!", 0);
}
// Send email to administrator if we run out of FOO
if (!($foo = allocate_new_foo())) {
error_log("Oh no! We are out of FOOs!", 1, "[email protected]");
}
?>
Enabling Error Logging in php.ini
PHP errors can be logged by opening the php.ini file and adding or uncommenting the lines of code below:
error_reporting = E_ALL & ~E_NOTICE
error_reporting = E_ALL & ~E_NOTICE | E_STRICT
error_reporting = E_COMPILE_ERROR|E_RECOVERABLE_ERROR|E_ER… _ERROR
error_reporting = E_ALL & ~E_NOTICE
Enabling PHP error logs in an individual file is simple. Just add the following lines of code at the beginning of the PHP file.
ini_set('display_errors', 1);
ini_set('display_startup_errors', 1);
error_reporting(E_ALL);
For parsing the log errors in the php.ini file, enable the following:
display_errors = on
The logged errors are displayed in the browser. You can also use the following commands to log PHP errors:
// Turn off all error reporting
error_reporting(0);
// Report simple running errors
error_reporting(E_ERROR | E_WARNING | E_PARSE);
// Reporting E_NOTICE can be good too (to report uninitialized variables or catch variable name misspellings ...)
error_reporting(E_ERROR | E_WARNING | E_PARSE | E_NOTICE);
// Report all errors except E_NOTICE
error_reporting(E_ALL & ~E_NOTICE);
// Report all PHP errors (see changelog)
error_reporting(E_ALL);
// Report all PHP errors
error_reporting(-1);
// Same as error_reporting(E_ALL);
ini_set('error_reporting', E_ALL);
Viewing PHP Logging Error
PHP error logging is turned off by default and can only be accessed when an error occurs. Before making any modifications to the code or configuration, you should first verify that logging is enabled in the PHP settings for your PHP hosting account.
1. Use the .htaccess File
Changing the .htaccess file enables the PHP error log. Using a file manager or FTP client, search for the .htaccess file in the website’s root directory. In case the file is hidden, you will need to change the settings in the control panel or your FTP client.
When the .htaccess file has been located, proceed as follows. In the .htaccess file, add this code:
php_flag log_errors on
php_value error_reporting 32767
php_value error_log “error_log.txt
- Under the public_html directory, create a file named error_log.txt.
- Close the file after saving.
- The error_log.txt file will contain all the PHP errors.
Local Value must now be active as error logging has been facilitated for PHP. Even when the Master Value is off, it would be overridden by the local value.
You can view the PHP error logs by opening the error_log.txt file if the website has any problems.
2. Use the php.ini File
One way to activate the PHP error log is by editing the php.ini file. It comes preconfigured web server that helps to run the PHP applications. The php.ini configuration file can be accessed through the PHP information. Use CTRL + F (Windows) or Command + F (Mac OS) to open up the search box. Look for “Loaded Configuration File.”

You can also make use of the command line to locate the configuration file. Use an SSH client for connecting to the web server. Run the following command:
php -i | grep php.ini
Following is the output:

Here are some directories for php.ini for various operating systems:
- For CLI: /etc/php/7.4/cli/php.ini. Modifications in the PHP configurations in CLI do not have any effect on the web server.
- For Nginx or Apache with PHP-FPM: /etc/php/7.4/fpm/php.ini
- For Apache: /etc/php/7.4/apache2/php.ini.
Copying PHP Error Logs to File
When working in a development setting, the above-described procedures are useful. But, once your site is live and you’re in production mode, you’ll need to hide the problems from the user interface and instead log them in a separate file. They should be kept in the PHP.ini-defined error logs location.
If PHP is configured to use an Apache2 module, the error logs will be kept in the /var/log/apache2 directory. PHP error log files are usually stored in your root directory’s /log subfolder on shared hosting. Nevertheless, if you have access to the php.ini file, you can change this:
error_log = /var/log/php-scripts.log
The master log file in cPanel is located at:
/usr/local/apache/logs/error_log
When nothing works, you can use the following:
<?php phpinfo(); ?>
Error logging in PHP Frameworks
You can use the above methods for core PHP development. However, many micro-frameworks and Model View Architecture (MVC) have been built on top of PHP’s fundamental functionality, each adding its own set of practices. CodeIgniter, Symfony, and Laravel are a few of the top PHP MVC frameworks for developing complex online applications.
In new Laravel projects, exception handling and error logging are already set up. Laravel’s App\Exceptions\Handler class is dedicated to handling such situations. Laravel uses the powerful Monolog library to log errors. It preconfigures several such handlers, offering the choice of using a PHP error log file, writing error information to the system log, and rotating log files.
Configuring the PHP option to display error messages to the user can be done in the config/app.php file. APP_DEBUG is an environment variable whose value can be adjusted in the.env file and then reflected in the app.php configuration. This value can be true during local development but must be false once migrated to production. An error message displayed in the browser indicates a potential security risk.
As a developer, you have the option of storing Laravel log information in a variety of formats, including an error log, Syslog, and the daily file. The app.php file is where you’ll make changes to the log option to configure Laravel error logging. For example, here’s how you’d go about establishing daily error logging:
‘log’ => ‘daily’
Errors accompanied by various security alerts can be documented in Monolog. It retains all errors by default, but you can classify them as emergency, error, critical, warning, or alert. To accomplish this effect, simply include the following attribute in your options:
‘log_level’ => env(‘APP_LOG_LEVEL’, ‘error’),
The log file can be found in storage/logs. Log facades can also be used to record errors.
<?php
namespace App\Http\Controllers;
use App\User;
use Illuminate\Support\Facades\Log;
use App\Http\Controllers\Controller;
class UserController extends Controller
{
/**
* Show the profile for the given user.
*
* @param int $id
* @return Response
*/
public function showProfile($id)
{
Log::info('Showing user profile for user: '.$id);
return view('user.profile', ['user' => User::findOrFail($id)]);
}
}
The logger provides eight different logging levels, including:
Log::emergency($message);
Log::alert($message);
Log::critical($message);
Log::error($message);
Log::warning($message);
Log::notice($message);
Log::info($message);
Log::debug($message);
Error Logging In Symfony
You can insert the default Symfony logger, which displays various warning levels, inside the controller. Log entries in the var/log/prod.log file are written to the var/log/dev.log file by default.
use Psr\Log\LoggerInterface;
public function index(LoggerInterface $logger)
{
$logger->info('I just got the logger');
$logger->error('An error occurred);
$logger->critical('I left the oven on!', [
// include extra "context" info in your logs
'cause' => 'in_hurry',
]);
// ...
}
The Laravel framework shares the same warning levels as its progenitor, Symfony.
For error logging, Symfony relies on Monolog and provides a default monolog.yaml with several pre-configured handlers. The following is an example of a log file that was written using syslogs:
// config/packages/prod/monolog.php
$container->loadFromExtension('monolog', [
'handlers' => [
'file_log' => [
'type' => 'stream',
'path' => '%kernel.logs_dir%/%kernel.environment%.log',
'level' => 'debug',
],
'syslog_handler' => [
'type' => 'syslog',
'level' => 'error',
],
],
]);
How to Automate the PHP Error Logging Process
While it is possible to manually report all failures, doing so can become tedious and time-consuming when working on many teams or large-scale projects. This is why it is important to implement a workflow that automatically records problems in third-party tools like Sentry, Blackfire, Slack, etc.
With these resources, you can learn more about problem causes and potential fixes. Slack channels can be set up to instantly alert everyone working on a project if something goes wrong during execution. Symfony is a good reference for a framework that follows a similar pattern.
How PHP errors are logged on Nestify
Your website’s server logs can provide information on PHP errors, including the URL of the requested page or file and the source of the request. Reviewing your server logs regularly can help you identify PHP errors and take action to fix them. At Nestify, you can access logs anytime from the Nestify dashboard. You can access logs in Manage Sites > Sites > Logs.
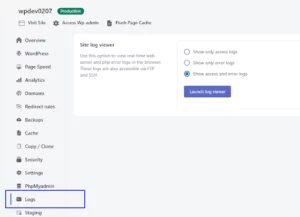
Conclusion
The PHP error log records information useful for debugging, such as the error message and the file and line number in the PHP code where it occurred. We hope this tutorial will be of great help to you to log your PHP errors both manually and automatically. Do let us know how you found this article and your queries below in the comment section.