In today’s digital age, social media integration has become crucial for websites to enhance user experience and streamline the login process.
One popular platform for integrating social logins is Facebook. By adding Facebook Login with a PHP Website, you can provide users with a convenient and secure way to sign in.
This article will guide you step-by-step on how to add Facebook Login with a PHP Website, ensuring a seamless user experience while increasing the credibility and trustworthiness of your website.
Benefits of Facebook Login
Facebook Login offers several benefits for both website owners and users. Incorporating Facebook login with a PHP website can:
- Simplify the registration and login process, reducing unease for users.
- Enable you to gather user data from their Facebook profiles, such as name, email, and profile picture.
- Increase user engagement and retention by personalizing the user experience based on their Facebook data.
- Enhance the security of your website by leveraging Facebook’s robust authentication system.
- Build trust and credibility as users perceive websites with social logins as more reputable.
Creating a Facebook App
To integrate Facebook Login into your PHP website, all you need to do is to create a Facebook app. Follow these steps to create your Facebook app:
- Log in to your Facebook account.
- Go to the Facebook Developers website and click on “My Apps” from the top navigation menu.
- Click on the “Create App” button.
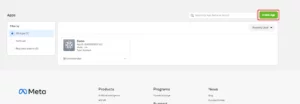
- Provide a quirky name for your app and select the desired app category.
- Finally, to continue, click the “Create App” button.
Obtaining the App ID and Secret Key
After creating your Facebook app, you need to obtain the App ID and Secret Key, which will be used in your PHP code. Here’s how you can obtain them:
- Go to the “Settings” tab of your Facebook app dashboard.
- Under the “Basic” settings, you will find your App ID.
- Click on the “Show” button next to the “App Secret” field to reveal your Secret Key. Copy it to a secure location.
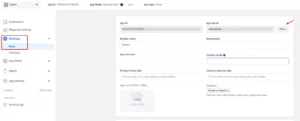
Installing the Facebook SDK for PHP
To seamlessly integrate the process, we will use the official Facebook SDK for PHP. Follow these steps to install the SDK:
- Download the Facebook PHP SDK from the official GitHub repository (https://github.com/facebook/php-graph-sdk).
- Extract the downloaded ZIP file to a location accessible by your PHP project.
- Include the autoload.php file from the SDK in your PHP script using the following code:
require_once ‘/path/to/facebook-php-sdk/autoload.php’;
Setting Up Facebook Login with a PHP Website
To securely enable Facebook Login with a PHP Website, follow these steps:
- Open your PHP file where you want to add the Facebook Login functionality.
- Initialize the Facebook SDK with your App ID and Secret Key using the following code:
$fb = new \Facebook\Facebook([
‘app_id’ => ‘your-app-id’,
‘app_secret’ => ‘your-app-secret’,
‘default_graph_version’ => ‘v12.0’,
]);
- Add the Facebook Login button to your HTML form by inserting the following code:
<a href=”<?php echo $fb->getRedirectLoginHelper()->getLoginUrl(‘https://your-website.com/fb-callback.php’, [’email’]); ?>”>Login with Facebook</a>
- Replace ‘https://your-website.com/fb-callback.php‘ with the URL of your callback script.
Handling the Facebook Login Response
Facebook will link users to your designated callback URL after they click the Facebook Login button and give permission. Take the following actions to respond to the response:
- Create a new PHP file (e.g., ‘fb-callback.php‘) to handle the Facebook login callback.
- Add the following code to the ‘fb-callback.php’ file to obtain the access token:
$helper = $fb->getRedirectLoginHelper();
$accessToken = $helper->getAccessToken();
- You can then use this access token to make API requests to retrieve user information.
Retrieving User Information
You can retrieve the user’s Facebook information, including their name and email, after acquiring the access token.
- Add the following code to your ‘fb-callback.php‘ file to retrieve the user’s Facebook profile:
try {
$response = $fb->get(‘/me?fields=name,email’, $accessToken);
$user = $response->getGraphUser();
$name = $user->getName();
$email = $user->getEmail();
} catch (\Facebook\Exceptions\FacebookResponseException $e) {
// Handle API errors
} catch (\Facebook\Exceptions\FacebookSDKException $e) {
// Handle SDK errors
}
- You can then use the retrieved user data to create or log in the user on your website.
Implementing User Logout
You can add a “Log out” button that commemorates the Facebook logout process for users to log out of your PHP website. To add this button, follow the below steps!
- Create a new PHP file (e.g., ‘logout.php‘) to handle the logout functionality.
- Add the following code to the ‘logout.php’ file to log out the user:
$helper = $fb->getRedirectLoginHelper();
$logoutUrl = $helper->getLogoutUrl($accessToken, ‘https://your-website.com’);
header(‘Location: ‘ . $logoutUrl);
exit();
- Replace ‘https://your-website.com‘ with the URL of your website’s homepage.
Customizing the Facebook Login Button
You can customize the appearance of the Facebook Login button to match your website’s design. Follow these steps:
- Wrap the Facebook Login button code with a div element and provide a unique ID:
<div id=”fb-login-button”>
<a href=”<?php echo $fb->getRedirectLoginHelper()->getLoginUrl(‘https://your-website.com/fb-callback.php’, [’email’]); ?>”>Login with Facebook</a>
</div>
- Add CSS styles to the div ID to customize the button’s appearance:
fb-login-button a {
/* Add your custom styles here */
}
Conclusion
By adding Facebook Login to your PHP website, you can provide a seamless and user-friendly login experience. Users can easily log in to your website using their Facebook credentials, while you gain access to their profile information.
Follow the step-by-step guide in this article to integrate Facebook Login with a PHP Website and enhance its user engagement, security, and credibility.
Troubleshooting and FAQs
Why am I getting an “Invalid App ID” error?
Make sure the App ID is entered correctly in your PHP code. Check again for any mistakes or missing characters.
How can I retrieve additional user information, such as profile picture or birthday?
Modify the ‘fields’ parameter in the API request to include the desired fields. For example:
$response = $fb->get(‘/me?fields=name,email,picture,birthday’, $accessToken);
Can I use Facebook Login on multiple pages of my PHP website?
Yes, you can add the Facebook Login with a PHP Website button to multiple pages. Just make sure to handle the login response and retrieve user information accordingly.
Q: Is it possible to log out the user from both Facebook and my website simultaneously?
No, logging out the user from your website does not log them out from their Facebook account. The Facebook logout button only terminates the user’s session on your website.
Q: Can I customize the permissions requested during Facebook Login?
The scope parameter in the login URL can be changed to request more permissions, yes. For instance, here is how to ask for the ‘user_friends’ permission:
$loginUrl = $helper->getLoginUrl(‘https://your-website.com/fb-callback.php’, [’email’, ‘user_friends’]);