If you’re passionate about PHP, this blog is tailor-made for you. This comprehensive tutorial will take you on a journey through the vast world of PHP libraries, revealing how these powerful tools elevate functionality and simplify the development process.
PHP, a widely-adopted scripting language, is the backbone of countless websites, with approximately 72.9% of all websites relying on it. The language’s extensive library ecosystem enables developers to tackle complex tasks efficiently, making it one of the most popular choices for web development worldwide.
In fact, if you use a content management system (CMS), PHP is likely running in the background, powering your website—whether or not you’ve ever written a single line of code yourself. This widespread use and the inherent flexibility of PHP have ensured its dominance in the industry for over a decade, with its market share remaining consistently strong.
What Are PHP Libraries?
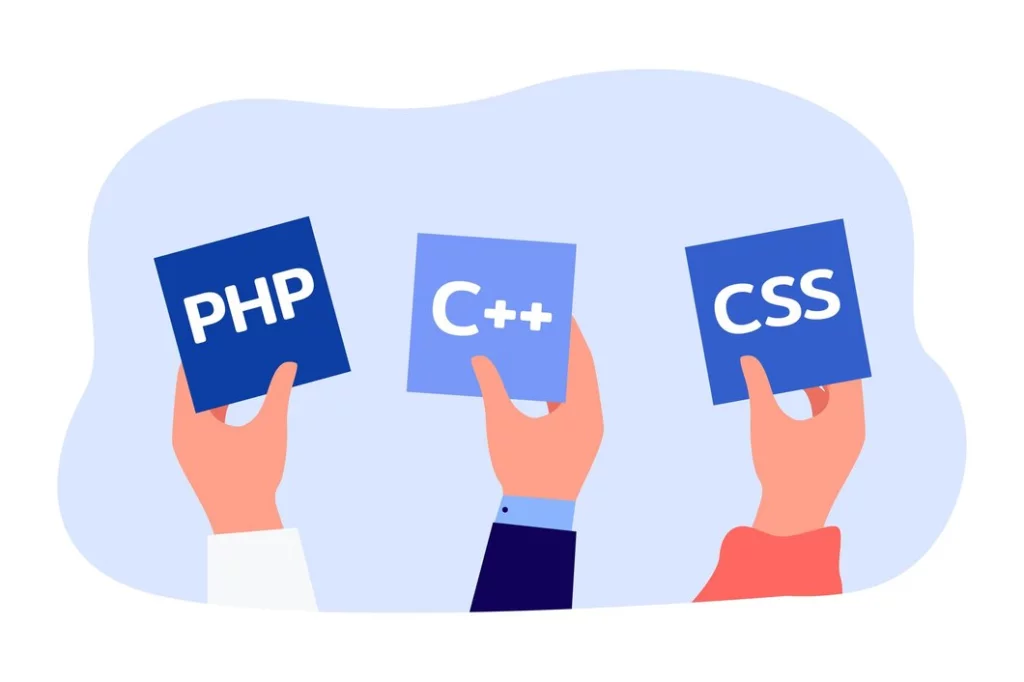
PHP libraries are collections of pre-written code that help developers simplify common tasks and functions, speeding up the development process. These libraries are essentially packages of code that developers can reuse across multiple projects.
A key example of PHP libraries is the Standard PHP Library (SPL), which is a set of classes and interfaces designed to address common programming challenges. These libraries offer predefined solutions that handle a wide range of tasks, from array manipulation to file handling, providing developers with tools to tackle common problems without starting from scratch.
By using PHP libraries, developers can integrate ready-made components into their applications, dramatically reducing the amount of custom code they need to write. This not only boosts productivity but also ensures that the development process is faster and more efficient.
Simplifying PHP Development: Why One Should Use PHP Libraries
1. Supercharge Your Efficiency
Time is precious in development, and PHP logging libraries help you reclaim it. Instead of reinventing the wheel with each project, these libraries offer ready-made solutions for common tasks, from file manipulation to form validation. With these tools at your disposal, developers can skip the repetitive coding and focus on building the core features of their applications. The result? A quicker turnaround, faster project delivery, and less time spent debugging.
2. Power of Community Collaboration
The beauty of PHP libraries, especially open-source ones, lies in the collective power of the global developer community. These libraries evolve and improve thanks to contributions from developers all around the world. As bugs get squashed and new features are added, the library becomes more refined and capable with each update.
Not only does this ensure you’re working with cutting-edge, reliable tools, but it also opens the door to community support, where you can learn from others, share solutions, and get assistance when needed.
3. Unlock a World of Extended Functionality
PHP libraries are like superchargers for PHP’s native capabilities. They extend the language far beyond its core functionalities, offering everything from advanced image manipulation to complex database interactions, and even creating PDFs with just a few lines of code.
These libraries empower developers to implement sophisticated features with minimal effort, enabling them to focus on the unique elements of their projects while leaving the heavy lifting to the library. With so many features at your fingertips, your applications can do so much more than you ever imagined.
4. Boost Security with Best Practices
Security is a critical concern in every PHP development project, and PHP logging libraries help safeguard your code. Many of the most popular libraries are built and maintained by experienced developers who prioritize security best practices.
These libraries are regularly updated to patch vulnerabilities and ensure that your applications stay protected against common threats like SQL injection, cross-site scripting (XSS), and other exploits. By relying on these trusted libraries, developers can rest easy knowing that their code adheres to industry-standard security protocols.
5. Seamless Scalability for Future Growth
Building scalable applications is essential for any growing project, and PHP libraries are designed with that in mind. Whether you’re working on a small blog or a massive enterprise-level platform, libraries help ensure that your application can grow alongside your user base, traffic, and data needs.
By tapping into these resources, developers can create flexible, high-performance applications that scale effortlessly, without compromising on speed or efficiency. With PHP libraries, you’re building for today and tomorrow.
Versatile PHP Libraries to Streamline Web Development Workflow
1. Guzzle HTTP Client
Guzzle is a HTTP client PHP develpment library that simplifies HTTP request sending and online service integration. It supports HTTP/1.1 and HTTP/2 and includes capabilities such as asynchronous request sending, handling redirects, and proxy support. Guzzle is commonly used by PHP developers to make API queries, and it interfaces with numerous popular APIs, such as Amazon Web Services, Google Maps, and Twitter.
Example:
use GuzzleHttp\Client;
$client = new Client();
$response = $client->request('GET', 'https://api.example.com');
echo $response->getStatusCode(); //200
echo $response->getBody(); //{"message": "Hello World!"}
2. Twig Template Engine
Twig is a modern and flexible template engine for PHP applications that allows developers to separate the presentation logic from the application logic in the web development process. To mitigate security vulnerabilities, it has template inheritance, sandbox mode, and automatic variable escaping. Symfony, Laravel, and Drupal are popular PHP frameworks that employ Twig extensively.
Example:
require_once 'vendor/autoload.php';
$loader = new \Twig\Loader\FilesystemLoader('templates');
$twig = new \Twig\Environment($loader);
echo $twig->render('index.html.twig', ['name' => 'John']);
3. PHPMailer
Popular PHP package PHPMailer enables PHP programs to send emails. Multiple recipients, attachments, HTML emails, and SMTP authentication are just a few of the features it offers. PHPMailer is easy to use and provides a wide range of setting options.
Example:
use PHPMailer\PHPMailer\PHPMailer;
$mail = new PHPMailer;
$mail->setFrom('[email protected]', 'From Name');
$mail->addAddress('[email protected]', 'To Name');
$mail->Subject = 'Email Subject';
$mail->Body = 'Email Body';
if(!$mail->send()) {
echo 'Message could not be sent.';
echo 'Mailer Error: ' . $mail->ErrorInfo;
} else {
echo 'Message has been sent';
}
4. Carbon Date and Time Library
Carbon are prevalent PHP libraries that permits the modification of dates and times in code quality. It offers an uncomplicated and resourceful API for interpreting and formatting dates and times, as well as handling several time zones.
Example:
use Carbon\Carbon;
echo Carbon::now()->format('Y-m-d H:i:s'); //2023-02-21 11:00:00
echo Carbon::parse('2023-02-21')->addDay(); //2023-02-22
5. Monolog Logging PHP Library
File, email, Syslog, and database handlers are just some of the preferences available with the Monolog PHP logging package. Log levels such as debug, info, warning, error, and critical are supported, and an adaptable and user-friendly API is provided to test data. For logging and debugging objectives, PHP applications and frameworks frequently employ Monolog.
Example:
use Monolog\Logger;
use Monolog\Handler\StreamHandler;
$log = new Logger('my_logger');
$log->pushHandler(new StreamHandler('logs/app.log', Logger::WARNING));
$log->warning('Warning message');
6. PHPUnit
PHPUnit is a PHP framework for unit testing that enables programmers to enroot automated tests for their code. It includes a miscellany of functions and tools for creating and running tests, making it easy to uncover faults and append code quality.
Example:
use PHPUnit\Framework\TestCase;
function add($a, $b) {
return $a + $b;
}
class AddTest extends TestCase {
public function testAdd() {
$this->assertEquals(4, add(2, 2));
}
}
7. Symfony Console
Symfony Console is a PHP module that adds a command prompt to PHP programs. (CLI). With Symfony Console, programmers can easily create CLI programs that respond to console input and demonstrate results to the user.
Example:
use Symfony\Component\Console\Application;
use Symfony\Component\Console\Command\Command;
use Symfony\Component\Console\Input\InputInterface;
use Symfony\Component\Console\Output\OutputInterface;
class HelloWorldCommand extends Command
{
protected static $defaultName = 'hello:world';
protected function execute(InputInterface $input, OutputInterface $output)
{
$output->writeln('Hello, World!');
return Command::SUCCESS;
}
}
$application = new Application();
$application->add(new HelloWorldCommand());
$application->run();
8. SwiftMailer
Application developers can send emails using SwiftMailer, a powerful PHP email framework. It offers attachments, several email transport options, and HTML email templates. SwiftMailer is now among indispensable PHP libraries for developers due to the community’s extensive use of it.
Example:
$transport = (new Swift_SmtpTransport('smtp.gmail.com', 587, 'tls'))
->setUsername('your_gmail_username')
->setPassword('your_gmail_password');
$mailer = new Swift_Mailer($transport);
$message = (new Swift_Message('Wonderful Subject'))
->setFrom(['[email protected]' => 'John Doe'])
->setTo(['[email protected]', '[email protected]' => 'A name'])
->setBody('Here is the message itself');
$result = $mailer->send($message);
9. Laravel Framework
Laravel is an estimable PHP framework that provides a great assortment of tools for developing custom-made applications and websites. Its minified syntax and compatibility with a variety of databases make it a meritable alternative for constructing cutting-edge online applications. Laravel has features specially designed for authentication, security, routing, and the Model-View-Controller framework.
Example:
To create a new route in Laravel, you can use the following code:
To create a new route in Laravel, you can use the following code:
Route::get('/welcome', function () {
return view('welcome');
});
10. Faker
Faker is a robust PHP module that helps programmers create counterfeited information that can be used for testing purposes. With the assistance of its many advantageous elements, creating realistic test data is a breeze for programmers.
Example:
To generate a random name using Faker, you can use the following code:
use Faker\Factory;
$faker = Factory::create();
echo $faker->name;
11. DOMDocument
A user interface for dealing with HTML and XML documents is facilitated with the power of DOMDocument module in the list of PHP libraries. It gives great versatility to programmers to decode, modify, and create XML and HTML pages. For web scraping and other data processing tasks, DOMDocument is progressively used by developers.
Example:
To load an HTML document using DOMDocument, you can use the following code:
$doc = new DOMDocument();
$doc->loadHTMLFile('https://example.com');
// Find all links in the document
$links = $doc->getElementsByTagName('a');
// Print the href attribute of each link
foreach ($links as $link) {
echo $link->getAttribute('href') . "\n";
}
12. Assert
Assert are among the PHP libraries that provides assertion functions for validating and testing code. It ensures that the code behaves as planned and catches mistakes before they reach production. Assert is simple to use and is compatible with common testing frameworks such as PHPUnit and Behat. To utilize Assert, it must be installed using Composer. Launch the terminal and navigate to the root directory of your project. Then, execute the subsequent command:
composer require webmozart/assert
Example:
Suppose we have a function that returns the sum of two numbers. Assert can be used to determine if a function behaves as intended.
use function Webmozart\Assert\Assert;
function add($a, $b) {
return $a + $b;
}
// Test the add() function
Assert::eq(add(2, 3), 5); // Passes
Assert::eq(add(-2, 3), 1); // Passes
Assert::eq(add(2, -3), -1); // Passes
Assert::eq(add(2, 3), 6); // Fails
Assert offers many other assertion functions, such as notEmpty(), greaterThan(), and contains(), among others.
13. Stripe PHP
Popular payment processing platform Stripe enables businesses to accept online payments. The PHP libraries like Stripe facilitates the integration of Stripe into PHP applications. To utilize Stripe, Composer installation is required. Launch the terminal and navigate to the root directory of your project. Then, execute the subsequent command:
composer require stripe/stripe-php
Example:
Let’s say we want to charge a customer for a product using Stripe.
require_once('vendor/autoload.php');
\Stripe\Stripe::setApiKey('sk_test_...');
// Create a new customer
$customer = \Stripe\Customer::create([
'email' => '[email protected]',
'source' => 'tok_visa'
]);
// Charge the customer
$charge = \Stripe\Charge::create([
'amount' => 1000,
'currency' => 'usd',
'customer' => $customer->id,
'description' => 'Charge for product'
]);
14. Omnipay
Omnipay is among the list of the best PHP libraries for payment processing that offers a consistent API for numerous payment gateways. It enables integration with numerous payment gateways using a single, standardized API. This article will examine Omnipay and provide an illustration of its application. To utilize Omnipay, it must be installed via Composer. Launch the terminal and navigate to the root directory of your project. Then, execute the subsequent command:
composer require omnipay/omnipay
This will install Omnipay and its dependencies.
Example:
Let’s say we want to process a payment using Omnipay with the Stripe payment gateway.
require_once('vendor/autoload.php');
use Omnipay\Omnipay;
$gateway = Omnipay::create('Stripe');
$gateway->setApiKey('sk_test_...');
$response = $gateway->purchase([
'amount' => '10.00',
'currency' => 'USD',
'description' => 'Purchase description',
'card' => [
'number' => '4242424242424242',
'expiryMonth' => '12',
'expiryYear' => '2022',
'cvv' => '123',
],
])->send();
if ($response->isSuccessful()) {
echo "Transaction was successful!\n";
echo "Transaction reference: " . $response->getTransactionReference() . "\n";
} else {
echo "Transaction failed: " . $response->getMessage() . "\n";
}
15. Spatie Image Optimizer
Spatie Image Optimizer are PHP libraries that enables image optimization for faster loading times and smaller file sizes. This post will examine Spatie Picture Optimizer and present an illustration of its application. To utilize Spatie Picture Optimizer, Composer installation is required. Launch the terminal and navigate to the root directory of your project. Then, execute the subsequent command:
composer require spatie/image-optimizer
This will install Spatie Image Optimizer and its dependencies.
Example:
Let’s say we have an image that we want to optimize.
require_once('vendor/autoload.php');
use Spatie\ImageOptimizer\OptimizerChainFactory;
$optimizerChain = OptimizerChainFactory::create();
$optimizerChain->optimize('path/to/image.jpg');
16. Minify
Minify are PHP libraries that enables you to minify your HTML, CSS, and JavaScript code for quicker page loads and smaller file sizes. This article will discuss Minify and provide an example of its application. To utilize Minify, Composer installation is required. Launch the terminal and navigate to the root directory of your project. Then, execute the subsequent command:
composer require mrclay/minify
This will install Minify and its dependencies.
Example:
Let’s say we have a CSS file that we want to minify.
require_once('vendor/autoload.php');
use MatthiasMullie\Minify\CSS;
$css = new CSS('path/to/styles.css');
$css->minify('path/to/minified.css');
17. Swap
Swap are flexible PHP libraries that allows text strings to be converted from one format to another. With Swap, it is simple to convert between HTML, plain text, and numerous more forms. To utilize Swap, it must be installed using Composer. Launch the terminal and navigate to the root directory of your project. Then, execute the subsequent command:
composer require florianv/swap
This will install Swap and its dependencies.
Example:
Let’s say we have a string in HTML format that we want to convert to plain text.
require_once('vendor/autoload.php');
use Swap\Converter;
$converter = new Converter();
$htmlString = "<h1>Hello, world!</h1><p>This is a paragraph.</p>";
$textString = $converter->convert($htmlString, 'html', 'text');
echo $textString;
18. Tcpdf
TCPDF is a powerful PHP library for creating PDF documents programmatically. TCPDF allows you to create PDF documents with text, graphics, and other elements. Launch the terminal and navigate to the root directory of your project. Then, execute the subsequent command:
composer require tecnickcom/tcpdf
This will install TCPDF and its dependencies.
Example:
Let’s say we want to create a simple PDF document that contains the text “Hello, world!”.
require_once('vendor/autoload.php');
use TCPDF;
$pdf = new TCPDF();
$pdf->AddPage();
$pdf->SetFont('times', 'BI', 20);
$pdf->Write(0, 'Hello, world!');
$pdf->Output('hello_world.pdf', 'D');
19. Sylius
Sylius is a PHP library for reinforcing custom ecommerce apps. A developer can design innovative products, shopping carts, and orders with Sylius. To utilize Sylius, it must be installed using Composer. Launch the terminal and navigate to the root directory of your project. Then, execute the subsequent command:
composer require sylius/sylius
This will install Sylius and its dependencies.
Example:
Let’s say we want to create a simple e-commerce application that allows users to purchase products.
require_once('vendor/autoload.php');
use Sylius\Bundle\CoreBundle\Test\Factory\TestCartItemFactory;
use Sylius\Bundle\CoreBundle\Test\Factory\TestOrderFactory;
use Sylius\Bundle\CoreBundle\Test\Factory\TestProductFactory;
use Sylius\Bundle\ResourceBundle\Doctrine\ORM\EntityRepository;
use Sylius\Component\Core\Model\OrderInterface;
use Sylius\Component\Core\Model\ProductInterface;
use Sylius\Component\Resource\Factory\FactoryInterface;
use Sylius\Component\Resource\Repository\RepositoryInterface;
// Create a new product
$productFactory = new TestProductFactory(new EntityRepository());
$product = $productFactory->create(['name' => 'Product', 'price' => 100]);
// Create a new cart item for the product
$cartItemFactory = new TestCartItemFactory(new FactoryInterface());
$cartItem = $cartItemFactory->create(['quantity' => 1, 'product' => $product]);
// Create a new order with the cart item
$orderFactory = new TestOrderFactory(new RepositoryInterface());
$order = $orderFactory->createNew();
$order->addItem($cartItem);
// Checkout the order
$order->setState(OrderInterface::STATE_NEW);
$order->completeCheckout();
// Save the order
$em = $orderFactory->getEntityManager();
$em->persist($order);
$em->flush();
20. OAuth 2.0
OAuth 2.0 is a standard that unravels users to authenticate and grant access to their data to third-party applications without revealing their login credentials. You can create secure authentication and authorization in your applications by using an OAuth 2.0 PHP library. To utilize an OAuth 2.0 PHP library, it must be installed using Composer. Launch the terminal and navigate to the root directory of your project. Then, execute the subsequent command:
composer require league/oauth2-client
This will install the OAuth 2.0 PHP library and its dependencies.
Example:
Let’s say we want to authenticate and authorize a user to access their Google Drive files.
require_once('vendor/autoload.php');
use League\OAuth2\Client\Provider\Google;
// Set up the Google OAuth 2.0 provider
$provider = new Google([
'clientId' => 'your_client_id',
'clientSecret' => 'your_client_secret',
'redirectUri' => 'https://your-redirect-uri',
]);
// If we don't have an authorization code, get one
if (!isset($_GET['code'])) {
// Fetch the authorization URL from the provider
$authUrl = $provider->getAuthorizationUrl();
// Redirect the user to the authorization URL
header('Location: '.$authUrl);
exit;
} else {
// If we have an authorization code, exchange it for an access token
$accessToken = $provider->getAccessToken('authorization_code', [
'code' => $_GET['code']
]);
// Use the access token to access the user's Google Drive files
$client = $provider->getAuthenticatedClient($accessToken);
$files = $client->get('https://www.googleapis.com/drive/v3/files')->getBody();
var_dump($files);
}
From Good to Great: How to Pick the Right PHP Library for Your Web Development
1. Understand Your Project’s Requirements
Before diving into the sea of PHP libraries, it’s essential to have a clear understanding of your project’s specific needs. What functionality does your project require? Do you need a library for data manipulation, image processing, user authentication, or something else?
Key Questions to Ask:
- What is the core functionality you need from the library? (e.g., form validation, file handling, or API requests)
- Are you building a small website or a large-scale enterprise application?
- What kind of performance requirements do you have? (e.g., high traffic handling, real-time interactions)
- Are there any specific security concerns your project faces?
Once you’ve answered these questions, you’ll have a clearer idea of what type of library will be most beneficial. For instance, if you need to handle file uploads securely, libraries like Flysystem can be very helpful. On the other hand, if you’re building a RESTful API, Guzzle or Symfony HttpClient might be better suited to your needs.
2. Evaluate Library Popularity and Community Support
One of the best indicators of a PHP library’s quality is its popularity. Libraries with a large user base tend to be more reliable, frequently updated, and well-documented. This is particularly important when you encounter issues or need support during development. A robust community ensures that bugs are quickly identified and resolved, and new features are regularly added.
How to Check Library Popularity:
- GitHub Stars & Forks: Check how many stars and forks the library has on GitHub. Popular libraries typically have a large number of stars, which reflects the number of developers who have found it useful.
- Issues and Pull Requests: A good library will have an active repository with issues being resolved promptly. Check the open and closed issues to gauge how well the library is maintained.
- Forum Discussions and Reviews: Look for community discussions on forums, blogs, or Stack Overflow. A strong community presence can give you confidence that the library will be reliable in the long run.
3. Check for Active Maintenance and Regular Updates
Libraries that are actively maintained are more likely to work with the latest versions of PHP and other dependencies. Libraries that haven’t been updated in a while might be incompatible with new PHP versions or may contain unresolved bugs and security vulnerabilities.
How to Ensure Active Maintenance:
- Release History: Look at the release history on the library’s GitHub repository. Regular commits and version updates are a good sign of an actively maintained library.
- Last Update Date: Check when the library was last updated. If it’s been more than a year without updates, it could be a sign that the library is no longer actively supported.
- Changelog and Roadmap: A well-documented changelog shows the library’s update history and new features. A clear roadmap signals future improvements, which is crucial for long-term reliability.
4. Review the Documentation
Good documentation is crucial for effectively using a library. A well-documented library can significantly speed up your development process by providing clear instructions, use cases, examples, and troubleshooting tips. Poor documentation, on the other hand, can lead to confusion, wasted time, and unnecessary errors.
What to Look for in Documentation:
- Installation Guides: Clear instructions on how to install and set up the library.
- Code Examples: Practical examples of how to use the library in real-world scenarios.
- API Documentation: If the library offers an API, check if it has detailed API documentation.
- Troubleshooting Section: A section for common errors and solutions can save a lot of time during development.
If the documentation is outdated or hard to follow, that’s a red flag. Opt for libraries that make it easy for you to understand their features and integrate them seamlessly into your project.
5. Assess the Library’s Performance
Performance is always a critical consideration, especially when developing large applications or handling high traffic volumes. Some libraries may introduce significant overhead, which can impact your app’s speed and scalability. Make sure that the library you choose performs efficiently for your specific use case.
Performance Tips:
- Benchmarking: Run performance tests to see how the library performs in real-world conditions. Compare its speed and memory usage against other libraries.
- Load Testing: For libraries that will be used in high-traffic scenarios (like caching or image processing), ensure that it can handle large loads without degrading performance.
- Scalability: Check if the library is designed to scale effectively. Some libraries may work fine on smaller projects but struggle when handling more data or users.
6. Compatibility with Other Tools and Frameworks
Consider the environment in which your project is being developed. Is it built on a specific PHP framework like Laravel, Symfony, or Zend? Does the library integrate well with other tools you’re using, such as database systems, front-end technologies, or other back-end services?
Key Compatibility Considerations:
- PHP Version Compatibility: Ensure that the library supports the version of PHP you’re using in your project.
- Framework Integration: Some libraries are built specifically to integrate with popular PHP frameworks. For example, Laravel has a dedicated ecosystem of libraries that work seamlessly with it, such as Laravel Collections or Laravel Horizon.
- Third-Party Integrations: If your project relies on third-party services (e.g., payment gateways, social media APIs), check that the library supports these integrations.
7. Security Considerations
Security should always be a top priority when choosing a PHP library. Libraries that are frequently updated and well-maintained are less likely to have security vulnerabilities. Look for libraries that follow best practices for security, especially when handling sensitive data like passwords, user information, or financial transactions.
Security Features to Consider:
- Data Validation & Sanitization: Libraries that include built-in functions for validating and sanitizing user input help protect against common security threats like SQL injection and XSS attacks.
- Encryption Support: If your project handles sensitive data, choose libraries that support strong encryption methods and secure data storage.
- Security Audits: Libraries that undergo regular security audits are more likely to be free from vulnerabilities. Check if the library’s maintainers have conducted security assessments or if they follow industry-standard practices.
8. Evaluate Licensing and Costs
Make sure the library you choose aligns with your project’s licensing needs. While many PHP libraries are open-source, some may come with restrictions or require a paid license for commercial use.
What to Check:
- Open-Source License: Ensure that the library is licensed under a permissive open-source license (such as MIT or GPL) if you’re working on a project that will be publicly released.
- Commercial Licensing: If you’re working on a commercial project, check if the library requires a commercial license or has limitations for commercial use.
- Costs: Some libraries may be free to use, while others might offer premium features under a paid model. Ensure that you’re clear on the costs involved, especially for larger-scale projects.
9. Test Before Full Integration
Once you’ve narrowed down your choices, it’s a good idea to test the library in a small prototype or proof-of-concept. This allows you to assess how well it integrates with your project and whether it meets your performance and functionality expectations before committing to it fully.
Testing Tips:
- Prototype or Sandbox: Set up a small test environment where you can experiment with the library’s features.
- Integration Testing: Ensure that the library integrates smoothly with your existing codebase, framework, and database.
- Error Handling: Test the library’s ability to handle errors gracefully and make sure it provides helpful error messages when something goes wrong.
PHP Libraries: The Secret to Smarter, Faster, and More Secure Development
The right PHP library can be a game-changer for your project, turning complex tasks into smooth, seamless processes. By evaluating key aspects like functionality, performance, and security, you ensure that your development workflow is efficient and your application is built on a solid foundation.
Ready to give your PHP projects the hosting they deserve? Try Nestify Hosting for free and unlock blazing-fast speeds, top-tier security, and expert support designed to make your PHP development experience effortless. Get started today and let Nestify power your next big idea!
FAQs to Supercharge Your Development Process
How do I test a PHP library before using it in my project?
To test a PHP library, create a small prototype or sandbox environment where you can experiment with the library’s features. Test its performance, compatibility with your code, and ease of integration. This will help you identify any issues or limitations before committing to it in your main project.
How can I ensure my PHP application is secure when using libraries?
To ensure security, choose well-maintained libraries that follow best practices for secure coding. Look for libraries that are frequently updated and reviewed for vulnerabilities. Additionally, always validate and sanitize user inputs, and make use of encryption methods provided by trusted libraries to protect sensitive data.