This is the second post in the series that will talk about JavaScript. In the previous post, I presented a historical context of the emergence of language and how it has evolved so far.
In this post, we will cover version 6 of ECMAScript, giving some examples of what has changed and what has come again. If you doubt the history of version 6, read the previous post in the series.
When I started writing the series, I intended to approach version 5 of the language, which is the most used version today. But this scenario is changing. Numerous new projects are being done using ES 6. In addition, I attended a seminar on this version, and I enjoyed the participation of those present.
So I decided to change the scope and approach version 6 so that this series serves as a reference and has a didactic value more according to the present day and those to come.
Where does ECMAScript 6 come from?
If you read the previous post in the series, you probably know that ES 6 derives indirectly from version 4. ES 4 was dropped in July 2008 because it had a considerable feature package. This would make acceptance of the new version a bit difficult since the change would be very disruptive. The syntax and functions changed, and the classes arrived, anyway. Everything that JavaScript had not already come in version 4.
The TC-39 decided to abandon ES 4, create a patch for version 3 that did more than three but less than 4, and split the large feature pack into two versions, which would become versions 6 and 7, But these were only available in June 2015, when the TC-39 approved ECMAScript 6.
What’s new?
The list of new features is enormous. So I will cite only the most important and most anticipated.
Classes and inheritance
Classes, along with inheritance, came to bring the concept of object orientation closer to the definition of language. This definition was inherited purely from Java.
class Animal { constructor(name) { this.name = name; } walk() { console.log(`${this.name} is walking.`); } } class Dog extends Animal { bark() { console.log(`${this.name} says 'Woof'!`); } } var dog = new Dog('Lorkar'); dog.walk(); // > Lorkar is walking. dog.bark(); // > Lorkar says 'Woof'!
Default parameters
It was inherited from Python and Perl and made a massive lack of language.
class Dog extends Animal { bark(msg='Woof') { console.log(`${this.name} says '${msg}'!`); } } var dog = new Dog('Lorkar'); dog.walk(); // > Lorkar is walking. dog.bark(); // > Lorkar says 'Woof'! dog.bark('Ouch'); // > Lorkar says 'Ouch'!
Generators
Generators are like functions that pause while returning a value that may have been preprocessed. If the generator is called again, it summarizes the execution from where it left off, preserving the entire scope.
function* range(start, end, step) { while (start < end) { yield start; start += step; } } for (let i of range(0, 10, 2)) { console.log(i); } // > 0 // > 2 // > 4 // > 6 // > 8
Promises
Already present in Frameworks as the Angular, promises come native to ES 6.
class Dog extends Animal { bark() { return new Promise(function (req, rej) { setTimeout(req, 1000); }); } } function barkHandler() { console.log('Woof!'); } var dog = new Dog('Lorkar'); dog.bark().then(barkHandler); // > Woof! after 1 sec
Template literals
Classical inherited Perl and Python functionality.
class Dog extends Animal { bark(msg='Woof') { console.log(`${this.name} says ${msg}!`); } }
Arrow functions
The syntactic reduction of anonymous functions. Besides size, they have another exciting feature. They preserve the scope of the object where they are declared. The standard parts of JavaScript have their content; therefore, this point is to the function itself and not to the thing.
let square = x => x * x; let add = (a, b) => a + b; let pi = () => 3.1415; console.log(square(5)); console.log(add(3, 4)); console.log(pi());
Scope Block
ES 5 is famous for not providing scope for variables in blocks. Only within functions did this happen. This made the programmer devise ways to get around the problems it caused. Declaring the variables with let, the scope is preserved in the block where the variable is declared, regardless of whether it is an if, a, for, or a function. If said with var, the variables remain with the old behavior.
var x = 0; for (let i = 0; i < 10; i++) { x += 10; } try { console.log(i); } catch(e) { console.log( 'i does not exist here!' ); }
De-structured assignment
Syntactic sugar for extraction of internal attributes to an Array or Object.
let [one, two] = [1, 2]; let {three, four} = {three: 3, four: 4}; console.log(one, two, three, four);
Iterators
Similar to in already used in objects, but for use in Arrays, Sets, and Maps.
let arr = [1, 2, 3, 4, 5]; let sum = 0; for (let v of arr) { sum += v; } console.log('1 + 2 + 3 + 4 + 5 =', sum);
Spread Operators
Synthetic sugar that structures or disassembles arrays to facilitate the passage of parameters and avoid the use of arguments in functions, for example. React goes even further and enables this feature to be used to de-structure objects.
function add(a, b) { return a + b; } let nums = [5, 4]; console.log(add(...nums));
Not yet fully supported.
The official definition of the TC-39 on ES 6 was made in June 2015. That’s why today’s browsers mostly do not fully support ES 6. Google Chrome 53 has 97% compatibility. So it’s possible to play with ES 6 on the Chrome console without worrying about something that is not supported.
A reasonably reliable compatibility table keeps the specifications of ES 6 updated, not only this version but also 7, among others. Note that Google Chrome, Microsoft Edge (yes, Edge!) And Node 6.5 are the most compatible application.
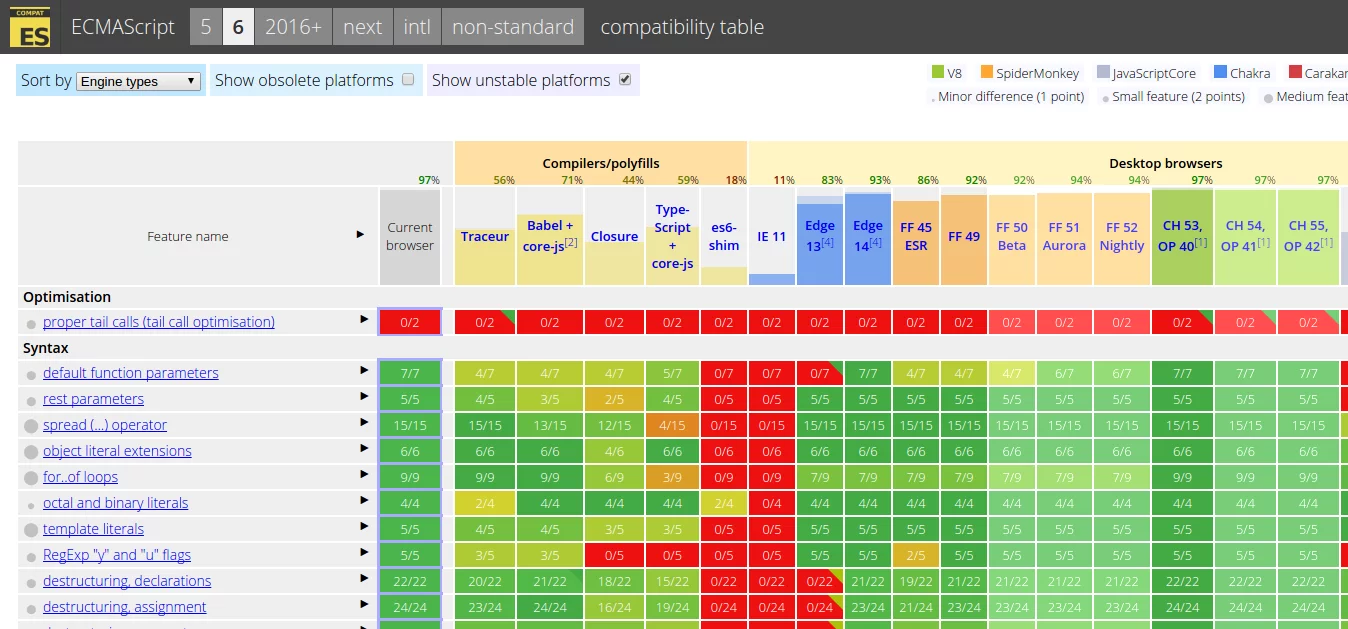
How can I use ES 6 today?
The answer is Babel!
Babel is a JavaScript compiler. Transcribing your ES 6 code with Babel will result in an ES 5 code. This is the best way to use ES 6 today without worrying about the environment where your code is running. In isolated environments and ensuring that you run on a compiler that supports ES 6, Babel is not required. An example of this is Node 6. x.
How is the ES 6 code transcribed for ES 5?
Here are some examples of what Babel does to simulate in ES 5 the behavior of the ES 6 features. These are two simple examples, only to show that the behavior is fully reproducible with version 5.
Note that all transcript codes use strict mode. Strict mode is enabled by default in ES 6.
Scope Block
// ES 6 var x = 0; for (let i = 0; i < 10; i++) { x += 10; } try { console.log(i); } catch(e) { console.log( 'I do not exist!' ); }
// Transcrito para ES 5 'use strict'; var x = 0; for (var _i = 0; _i < 10; _i++) { x += 10; } try { console.log(i); } catch (e) { console.log('i não existe aqui!'); }
Arrow functions
// ES 6 let square = x => x * x; let add = (a, b) => a + b; let pi = () => 3.1415; console.log(square(5)); console.log(add(3, 4)); console.log(pi());
//Transcrito para ES 5 "use strict"; var square = function square(x) { return x * x; }; var add = function add(a, b) { return a + b; }; var pi = function pi() { return 3.1415; }; console.log(square(5)); console.log(add(3, 4)); console.log(pi());
If you are curious about how other Babel-transcribed codes are, try to get the code from one of the examples above and play it on trying it out of Babel.
Babel uses presets to do the transcription. To transcribe code in ES 6, Babel uses the preset es2015. For ES 7 and React, for example, one can use the presets stage-2e react. This makes Babel extensible, so you can run JavaScript in the latest specs.
Things easy to do with ES 6 those were horrible to do with ES 5
Here are two examples of simple things to do with ES 6, which yielded a veritable dribble in the language (and consequently a lot of code) with ES 5.
Classes
Of course, the best example! Classes do not exist in ES 5. To simulate this behavior, a primary function (a self-executing closure) was declared to serve as an initializer. Then it was said a constructor function that received functions and attributes in the prototype. See the example in ES 5:
// ES 5 // Declarando var Animal = (function () { function Animal(name) { // Função construtura this.name = name; } Animal.prototype.walk = function walk() { console.log(this.name + ' is walking!'); } return Animal; // Esse é o animal do closure. Não o de fora. }()) // <= Auto executável // Usando var animal = new Animal('Lorkar'); animal.walk(); // > 'Lorkar is walking!'
The equivalent in ES 6
// ES 6 // Declarando class Animal { constructor(name) { this.name = name; } walk() { console.log(`${this.name} is walking!`); } } // Usando let animal = new Animal('Lorkar'); animal.walk(); // > 'Lorkar is walking!'
Notice that the way to use it is the same. But the statement is frighteningly different. And we can also take advantage of a literal template in the function phrase walk. ES 6 came with the classes to make things easier.
I will not present an example with inheritance here because the code in ES 5 is pervasive. A simple salad of code to do something in ES 6 is done with one class Animal extends MyObject.
Scope Block
In ES 5, the variables do not have block scope. The only thing that gives coverage to the variables is the functions. Therefore, it was common to see blocks encompassed by a primary role to protect the variable’s scope. See the example in ES 5.
// ES 5 for (var i = 0; i < 10; i++) { console.log(i); } // > 0 // > ... // > 9 console.log(i); // > 9 (function () { for (var i = 0; i < 10; i++) { console.log(i); } }()) // > 0 // > ... // > 9 console.log(i); // > Uncaught ReferenceError: i is not defined
The equivalent in ES 6:
// ES 6 for (var i = 0; i < 10; i++) { console.log(i); } // > 0 // > ... // > 9 console.log(i); // > 9 for (let i = 0; i < 10; i++) { console.log(i); } // > 0 // > ... // > 9 console.log(i); // > Uncaught ReferenceError: i is not defined
Notice the use of let. If the variables are declared with let, the scope will be preserved within the block {}.
Conclusion
ES 6 came to fix the house on the issue of syntax and features. As seen in the two examples above, what was a nightmare to do in ES 5 became simple to do in ES 6.
In addition, the classes finally arrived and gave even more power to the language without taking advantage of the functions, which gained the arrow functions to make them even more powerful.
Did you like ES 6? Already developed using the technology? Tell us your experiences in the comments.